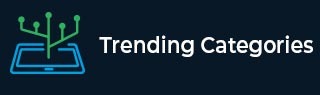
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Heaters in Python
Suppose we have to design a standard heater with a fixed warm radius to warm all the houses. Now, we have given positions of houses and heaters on a horizontal line, we have to find the minimum radius of heaters so that all houses could be covered by those heaters. So, we will provide houses and heaters separately, and our expected output will be the minimum radius standard of heaters.
So, if the input is like [1,2,3,4],[1,4], then the output will be 1 as the two heaters was placed in position 1 and 4. We have to use radius 1, then all the houses can be warmed.
To solve this, we will follow these steps −
sort the list houses
sort the list heaters
res := an array of size same as houses array, and fill this using inf
for i in range 0 to size of houses, do
h := houses[i]
ind := left most index to insert h into heaters so that list remains sorted
if ind is same as size of heaters, then
res[i] := minimum of res[i], |h - heaters[-1]|
otherwise when ind is same as 0, then
res[i] := minimum of res[i], |h - heaters[0]|
otherwise,
res[i] := minimum of res[i], |h - heaters[ind]| , |h - heaters[ind-1]|
return maximum of res
Example
Let us see the following implementation to get a better understanding −
from bisect import bisect_left class Solution: def findRadius(self, houses, heaters): houses.sort() heaters.sort() res = [float('inf')]*len(houses) for i in range(len(houses)): h = houses[i] ind = bisect_left(heaters, h) if ind==len(heaters): res[i] = min(res[i], abs(h - heaters[-1])) elif ind == 0: res[i] = min(res[i], abs(h - heaters[0])) else: res[i] = min(res[i], abs(h - heaters[ind]), abs(h - heaters[ind-1])) return max(res) ob = Solution() print(ob.findRadius([1,2,3,4],[1,4]))
Input
[1,2,3,4],[1,4]
Output
1