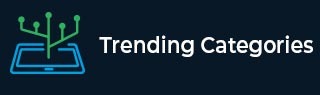
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Heap and Stack Memory Errors in Java
In Java, every interface, class, object, variable and method of a running program is stored in distinct reasons of computer memory. The heap is the part of memory area where values of variables, methods and classes are stored at runtime. Its allocation happens dynamically and can grow or shrink depending on the application's needs. On the other hand, the reference variables, names of methods and classes are stored in the stack memory area. However, if for some reason their allocation is not handled properly then, it may lead to memory errors that we are going to discuss in this article.
Error related to Stack
Whenever a process starts it automatically gets defined with a fixed stack size. Duringeach method call, a call frame gets created on the call stack and it continues until method invocation ends. When there is no space left for a new stack frame in the stack space of computer memory then we encounter StackOverflowError.
Example 1
The following example illustrates the StackOverflowError
import java.lang.StackOverflowError; public class Overflw { public static void methodA(int n1) { n1++; methodB(n1); } public static void methodB(int n1) { n1++; methodA(n1); } public static void main(String []args) { int n1 = 0; methodA(n1); } }
Output
Exception in thread "main" java.lang.StackOverflowError at Overflw.methodB(Overflw.java:10) at Overflw.methodA(Overflw.java:6) at Overflw.methodB(Overflw.java:10) at Overflw.methodA(Overflw.java:6) at Overflw.methodB(Overflw.java:10) at Overflw.methodA(Overflw.java:6) at Overflw.methodB(Overflw.java:10)
As you can see the output of example 1 code we are getting StackOverflowError. Here, we have created two parameterized user defined methods named ‘methodA’ and ‘methodB’.In the main method, we have declared and initialized an integer variable ‘n1’ to 0 and called the ‘methodA’ along with an argument ‘n1’. Now, the ‘methodA’ called the ‘methodB’ with the incremented value of ‘n1’. Again, the ‘methodB’ called the ‘methodA’ and this process repeats itself many times. Hence at some point, the stack size created for this program gets exhausted resulting in the following error.
The following measures we can take to handle StackOverflowError
Give proper termination conditions for methods that repeat themselves
Reducing the size of local variables or arrays may also help.
Refactor the code to avoid infinite method invocation.
Example 2
Now, with the help of this example we will try to find the solution for StackOverflowError occurred in the earlier example.
public class Overflw { public static void methodA(int n1) { n1++; methodB(n1); } public static void methodB(int n1) { n1++; int n2 = 5; int mult = n1 * n2; System.out.println("Value of n1 and n2 multiplication is: " + mult); } public static void main(String []args) { int n1 = 0; methodA(n1); } }
Output
Value of n1 and n2 multiplication is: 10
In example 1 the problem with the code was that lines 6 and 10 didn’t get terminated. Butin the above example, we have given a statement that terminates the program and printsthe value stored in ‘mult’.
Error related to Heap
The two attributes named ‘-Xmx’ and ‘-Xms’ of JVM determine the size of Heap. This size impacts the storage of values. When JVM faces a problem in the allocation of values due to insufficient space in the heap memory part then we encounter OutOfMemoryError
Example 1
The following example illustrates the OutOfMemoryError.
public class MemoryErr { public static void main(String[] args) { String stAray[] = new String[100 * 100 * 100000]; } }
Output
Exception in thread "main" java.lang.OutOfMemoryError: Java heap space at MemoryErr.main(MemoryErr.java:3)
In the above code, we have allocated a size that is greater than the heap size therefore,we got the OutOfMemoryError
The following measures we can take to handle OutOfMemoryError.
We can increase the size of heap using the -Xmx and -Xms JVM options
Using a garbage collector that suits the application's behavior may also help.
Example 2
The following example illustrates how we can handle the OutOfMemoryError using try and catch block.
public class MemoryErr { public static void main(String[] args) { try { String stAray[] = new String[100 * 100 * 100000]; } catch(OutOfMemoryError exp) { System.out.println("Application reached Max size of Heap"); } } }
Output
Application reached Max size of Heap
Conclusion
We started this article by explaining the two memory spaces required by each Java program. In the later sections, we have discussed the errors associated with the Heap and Stack memory