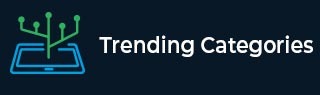
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Haskell Program to Print Hollow Right Triangle Star Pattern
In Haskell we can use the replicate function and recursive function to create a hollow right triangle star pattern.
A hollow right triangle star pattern is a pattern made up of asterisks (*) that forms a right triangle shape with empty spaces in the middle as shown below.
** * * * * * * * * * * * * ********
The shape is created by printing asterisks in a specific order, with the number of asterisks in each row increasing as the row number increases.
Algorithm
Step 1 − The printRow function is defined using the replicate/recursion function,
Step 2 − Program execution will be started from the main function. The main() function has whole control of the program. It is written as main = do. In the main function, a number is passed up to which the hollow right triangle star pattern is to be printed.
Step 3 − The variable named, “n” is initialized. It will hold the integer up to which the hollow right triangle star pattern is to be printed.
Step 4 − The result is printed to the console using the ‘putStrLn’ statement after the function is called.
Example 1
In this method, a function printPattern is defined that takes an integer n as an argument and returns a string representing a hollow right triangle star pattern of size n. The helper function printRow takes two integers n and i as arguments and returns a string representing a row of asterisks and spaces. The printRow function uses an if statement to determine whether the row should be filled with asterisks or whether it should be a hollow row with asterisks on the ends and spaces in the middle. The printPattern function uses list comprehension to generate n rows of asterisks and spaces.
module Main where printRow :: Int -> Int -> String printRow n i = if i == n then replicate i '*' ++ "
" else "*" ++ replicate (i - 1) ' ' ++ "*
" printPattern :: Int -> String printPattern n = concat [printRow n i | i <- [1..n]] main :: IO () main = putStr (printPattern 8)
Output
** * * * * * * * * * * * * ********
Example 2
In this example, the function is defined using the replicate function to print the hollow right triangle star pattern.
module Main where printRow :: Int -> Int -> String printRow n i = if i == 1 then "*
" else "*" ++ replicate (2 * i - 3) ' ' ++ "*
" printPattern :: Int -> String printPattern n = concat [printRow n i | i <- [1..n]] main :: IO () main = putStr (printPattern 8)
Output
* * * * * * * * * * * * * * *
Example 3
In this method, printRow is a recursive function that takes two arguments n and i representing the size of the pattern and the current row, respectively. If i is equal to 1, it returns a string with a single asterisk followed by a newline character. Otherwise, it returns a string with the first and last characters being asterisks and the middle part being a number of spaces equal to 2 * i - 3 (the number of spaces decreases as i increases), followed by a recursive call to printRow with i - 1 as the updated row number.
module Main where printRow :: Int -> Int -> String printRow n i | i == 1 = "*
" | otherwise = "*" ++ replicate (2 * i - 3) ' ' ++ "*
" ++ printRow n (i - 1) printPattern :: Int -> String printPattern n = printRow n n main :: IO () main = putStr (printPattern 8)
Output:
* * * * * * * * * * * * * * *
Conclusion
In Haskell, to print the hollow right triangle star pattern we can use replicate and recursive functions.