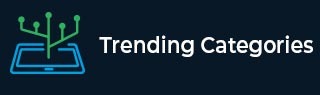
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Haskell Program to convert double type variables to int
In Haskell, we will convert double type variables into int by using user-defined function, doubleToInt along with floor, round and ceiling functions. In the first example, we are going to use (doubleToInt d = floor d) function and in the second example, we are going to use (doubleToInt d = round d). And in the third example, we are going to use (doubleToInt d = ceiling d).
Algorithm
Step 1 − The doubleToInt function is defined using floor function as, doubleToInt d = floor d.
Step 2 − The program execution will be started from main function. The main() function has whole control of the program. It is written as main = do.
Step 3 − The variable named, “d” is being initialized. It will hold the double value that is to be converted to respective integer value.
Step 4 − The function, doubleToInt is being called and d is passed to it.
Step 5 − The resultant integer value is printed to the console using ‘putStrLn’ statement after the function is called.
Example 1
In this example, a function doubleToInt is defined that takes a Double value d and returns an Int value by using the floor function to round down to the nearest integer. It then defines a main function that calls doubleToInt with a Double value and prints out both the original Double value and the resulting Int value.
doubleToInt :: Double -> Int doubleToInt d = floor d main :: IO () main = do let d = 3.14159 i = doubleToInt d putStrLn $ "The value of d is " ++ show d putStrLn $ "The value of i is " ++ show i
Output
The value of d is 3.14159 The value of i is 3
Example 2
In this example, the round function rounds the Double value to the nearest integer, and returns it as an Integral type, which can be directly converted to an Int.
doubleToInt :: Double -> Int doubleToInt d = round d main :: IO () main = do let d = 3.14159 i = doubleToInt d putStrLn $ "The value of d is " ++ show d putStrLn $ "The value of i is " ++ show i
Output
The value of d is 3.14159 The value of i is 3
Example 3
In this example, the ceiling function rounds the Double value up to the nearest integer, and returns it as an Integral type, which can be directly converted to an Int.
doubleToInt :: Double -> Int doubleToInt d = ceiling d main :: IO () main = do let d = 3.14159 i = doubleToInt d putStrLn $ "The value of d is " ++ show d putStrLn $ "The value of i is " ++ show i
Output
The value of d is 3.14159 The value of i is 4
Conclusion
Double to int conversion is the process of converting a Double data type to an Int data type. Double is a floating-point data type that can hold decimal values with high precision, while Int is an integer data type that can hold whole numbers only. In Haskell, an Int variable is converted to double using user-defined doubleToInt function along with floor, round and ceiling functions.