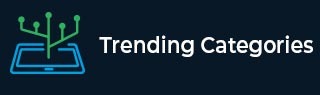
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Guess Number Higher or Lower II in C++
Suppose we are playing the Guess Game. The rules of the game is as follows −
- Player1 pick a number from 1 to n. player2 have to guess which number is picked by player1.
- Every time player2 guess wrong, player1 will tell whether the number that is picked is higher or lower.
However, when a player guess a particular number x, and another player guess wrong, another player has to pay $x. The game will end, when player2 got the correct answer.
For example if n = 10, and the player1 has taken 8
- In the first round, player2 tells the number is 5, that is wrong, actual number is higher, then he will give $5
- In the second round, player2 tells the number is 7, that is wrong, actual number is higher, then he will give $7
- In the third round, player2 tells the number is 9, that is wrong, actual number is lower, then he will give $9
Now the game ends. So total money given is $5 + $7 + $9 = $21.
To solve this, we will follow these steps −
- create one method called cost, that is taking low, high, another table dp
- if low >= high, return 0
- if dp[low, high] is not -1, then return dp[low, high]
- ans := inf
- for i in range low to high
- ans := min of ans and (i + max of cost(low, i – 1, dp) and cost(i + 1, high, dp))
- dp[low, high] := ans and return ans
- The actual method will be like −
- make one 2d array called dp of size (n + 1) x (n + 1), and fill this with -1
- return cost(1, n, dp)
Let us see the following implementation to get better understanding −
Example
#include <bits/stdc++.h> using namespace std; class Solution { public: int cost(int low, int high, vector < vector <int> >& dp){ if(low >= high)return 0; if(dp[low][high] != -1)return dp[low][high]; int ans = INT_MAX; for(int i = low; i <= high; i++){ ans = min(ans, i + max(cost(low, i - 1, dp), cost(i + 1, high, dp))); } return dp[low][high] = ans; } int getMoneyAmount(int n) { vector < vector <int> > dp(n + 1, vector <int> (n + 1, -1)); return cost(1, n, dp); } }; int main() { Solution ob1; cout << ob1.getMoneyAmount(8) << endl; return 0; }
Input
8
Output
12
Advertisements