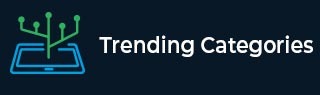
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Grouping an Array and Counting items creating new array based on Groups in JavaScript
Suppose, we have an array of objects like this −
const arr = [ { region: "Africa", fruit: "Orange", user: "Gary" }, { region: "Africa", fruit: "Apple", user: "Steve" }, { region: "Europe", fruit: "Orange", user: "John" }, { region: "Europe", fruit: "Apple", user: "bob" }, { region: "Asia", fruit: "Orange", user: "Ian" }, { region: "Asia", fruit: "Apple", user: "Angelo" }, { region: "Africa", fruit: "Orange", user: "Gary" } ];
We are required to write a JavaScript function that takes in one such array. The function should prepare a new array of objects that groups the data based on the "area" property of objects.
The function should also keep a count of the unique users for a particular area.
Therefore, for the above array, the output should look like −
const output = [ { "region": "Africa", "count": 2 }, { "region": "Europe", "count": 2 }, { "region": "Asia", "count": 2 } ];
Example
The code for this will be −
const arr = [ { region: "Africa", fruit: "Orange", user: "Gary" }, { region: "Africa", fruit: "Apple", user: "Steve" }, { region: "Europe", fruit: "Orange", user: "John" }, { region: "Europe", fruit: "Apple", user: "bob" }, { region: "Asia", fruit: "Orange", user: "Ian" }, { region: "Asia", fruit: "Apple", user: "Angelo" }, { region: "Africa", fruit: "Orange", user: "Gary" } ]; const groupByArea = (arr = []) => { const res = []; arr.forEach(el => { let key = [el.region, el.user].join('|'); if (!this[el.region]) { this[el.region] = { region: el.region, count: 0 }; res.push(this[el.region]); }; if (!this[key]) { this[key] = true; this[el.region].count++; }; }, Object.create(null)); return res; } console.log(groupByArea(arr));
Output
And the output in the console will be −
[ { region: 'Africa', count: 2 }, { region: 'Europe', count: 2 }, { region: 'Asia', count: 2 } ]
Advertisements