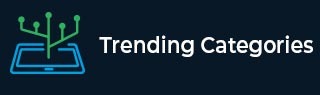
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Group a JavaScript Array
Suppose, we have a JavaScript array like this −
const data = [ { "dataId": "1", "tableName": "table1", "column": "firstHeader", "rows": [ "a","b","c" ] }, { "dataId": "2", "tableName": "table1", "column": "secondHeader", "rows": [ "d","e","f", ] }, { "dataId": "3", "tableName": "table2", "column": "aNewFirstHeader", "rows": [ 1,2,3 ] } ];
We are required to write a JavaScript function that in one such array. The function should construct a new grouped array of objects, based on the original array.
The grouped array should contain the data for separate, unique "tableName" objects into their own objects.
Therefore ultimately, the output should look like −
const output = [ { "tableName": "table1", "column": ["firstHeader", "secondHeader"], "rows": [["a","b","c"], ["d","e","f"]] }, { "tableName": "table2", "column": ["aNewFirstHeader"], "rows": [[1,2,3]] } ];
Example
const arr = [ { "dataId": "1", "tableName": "table1", "column": "firstHeader", "rows": [ "val","b","c" ] }, { "dataId": "2", "tableName": "table1", "column": "secondHeader", "rows": [ "d","e","f", ] }, { "dataId": "3", "tableName": "table2", "column": "aNewFirstHeader", "rows": [ 1,2,3 ] } ]; const groupArray = (arr = []) => { const res = arr.reduce((obj => (acc, val) => { let item = obj.get(val.tableName); if (!item) { item = { tableName: val.tableName, column: [], rows: [] } obj.set(val.tableName, item); acc.push(item); }; item.column.push(val.column); item.rows.push(val.rows); return acc; })(new Map), []); return res; }; console.log(JSON.stringify(groupArray(arr), undefined, 4));
Output
And the output in the console will be −
[ { "tableName": "table1", "column": [ "firstHeader", "secondHeader" ], "rows": [ [ "val", "b", "c" ], [ "d", "e", "f" ] ] }, { "tableName": "table2", "column": [ "aNewFirstHeader" ], "rows": [ [ 1, 2, 3 ] ] } ]
Advertisements