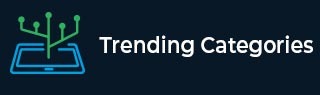
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Google Authentication in Django
In many developer websites, we get to see Google social authentication which is very handy. In this article, we will see how to create a Django Google login project.
First, go to https://console.cloud.google.com/apis/dashboardand create a project .
Go to Oauth consent screen and create a screen and save & continue.
Go to credentials and click "create credentials" and then "OAuth client ID":
Then, select web application, and add these two URLs −
- http://127.0.0.1:8000/
- http://127.0.0.1:8000/accounts/github/login/callback/
Now, you will get a client ID and a secret key, keep them safe in your file.
Example
Create a Django project and an app.
In settings.py −
SITE_ID = 1 LOGIN_REDIRECT_URL = "/" INSTALLED_APPS = [ 'django.contrib.admin', 'django.contrib.auth', 'django.contrib.contenttypes', 'django.contrib.sessions', 'django.contrib.messages', 'django.contrib.staticfiles', "django.contrib.sites", # <-- "allauth", # <-- "allauth.account", # <-- "allauth.socialaccount", # <-- "allauth.socialaccount.providers.google", "googleauthentication" #this is my app name ,you can name your app anything you want ] SOCIALACCOUNT_PROVIDERS = { 'google': { 'SCOPE': [ 'profile', 'email', ], 'AUTH_PARAMS': { 'access_type': 'online', } } } #add this in the end of file AUTHENTICATION_BACKENDS = ( "django.contrib.auth.backends.ModelBackend", "allauth.account.auth_backends.AuthenticationBackend", )
Here, we defined a Redirect URL. In INSTALLED APPs, we defined important backends that we are going to use for authentication. Then, we defined the social account provider which will tell what we should use for login (here we are using Google). We also defined what data of user should it store.
Now, in project's urls.py, add the following −
from django.contrib import admin from django.urls import path,include urlpatterns = [ path('admin/', admin.site.urls), path("accounts/", include("allauth.urls")), #most important path('',include("googleauthentication.urls")) #my app urls ]
Here, we added the default path that is needed to add; it is allauth library path for enabling Google login. The second one is app path that we made.
In app's urls.py −
from django.urls import path from . import views urlpatterns = [ path('',views.home), ]
Here, we set up our urls and rendered our views on home url.
In view.py −
from django.shortcuts import render # Create your views here. def home(request): return render(request,'home.html')
We simply rendered the frontend here.
Create a template folder in app's main directory and add a file home.html with the following content −
<!DOCTYPE html> <html> <head> <title>Google Registration</title> </head> <body> {% load socialaccount %} <h1>My Google Login Project</h1> <a href="{% provider_login_url 'google'%}?next=/">Login with Google</a> </body> </html>
Here, we rendered the JS and loaded the allauth library on frontend. In <a> we provided the Google login page on which we set up our default Google login page.
Now, run the following commands on the terminal −
python manage.py makemigrations python manage.py migrate
Next, create a superuser.
python manage.py createsuperuser
Then, start the server and go to admin panel. Go to Sites and add a site with url name and display name: http://127.0.0.1:8000
Go to Social applications and add application. Choose the site you added before −
This will register Google on your Django project as an authentication backend. Everything is set, now you can proceed to check the output.