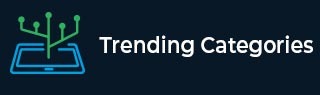
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Golang program to show use of super keyword in class
In golang, the "super" term doesn't exist in Go. The "super" keyword is used in object-oriented programming languages to denote the parent class or the class that is being inherited from. It is often used to access parent class methods or properties that the child class has modified. In this article, we will see how to use super keyword in the class with different examples.
Method 1: Using child struct and parent struct
In this Method, we will learn how to use super keyword in class using child struct and parent struct. Here, a Parent struct is embedded in the Child struct. Additionally, the Child struct contains a PrintName function that uses the "child.Parent.PrintName()" line to call the PrintName method of the Parent struct after printing its own name. Let’s see the execution.
Algorithm
Step 1 − Create a package main and declare fmt(format package) package in the program where main produces executable Example:s and fmt helps in formatting input and output.
Step 2 − Create a struct called "Parent" and give it a single string-type field called "name."
Step 3 − Create a method called "PrintName" on the Parent struct, with the receiver being a pointer to the struct. The "name" field's value is printed by the procedure.
Step 4 − Create a "Child" struct that embeds the Parent struct. A further field of type int called "age" is also present in the Child struct.
Step 5 − Create a method called "PrintName" on the Child struct, with the receiver being a pointer to the struct. The "name" field of the Child struct's value is printed first by the procedure. The "child.Parent.PrintName()" line is then used to invoke the "PrintName" function of the embedded Parent struct.
Step 6 − Create a pointer to a Child struct and fill its fields with values in the "main" method.
Step 7 − To use the "super" keyword, call the "PrintName" function on the Child struct pointer.
Step 8 − The output will be the child and parent’s name printed on the console using fmt.Println() function where ln means new line.
Example
In this example, we are going to use child struct and parent struct to show use of super keyword in class in Golang.
package main import "fmt" type Parent struct { name string //create name in the parent struct which will be used to print the name of parent } func (parent *Parent) PrintName() { fmt.Println("Parent:", parent.name) //print parent name } type Child struct { Parent age int } func (child *Child) PrintName() { fmt.Println("Child:", child.name) //print child name child.Parent.PrintName() // calling the PrintName method of the parent } func main() { child := &Child{Parent: Parent{name: "vipul kukreja"}, age: 16} child.PrintName() //call the method PrintName for child }
Output
Child: vipul kukreja Parent: vipul kukreja
Method 2 : Using rectangle struct and square struct
In this illustration, we will see how to use super keyword using rectangle struct and square struct. Here, an interface "Shape" with an "Area" method has been developed. This interface is implemented by the "Rectangle" struct, which has a method with the same signature. The "Square" struct has a "Area" function in addition to embedding the "Rectangle" type. The "sq.Rectangle.Area()" line is used to invoke the "Area" method of the embedded "Rectangle" struct from the "Area" method of the "Square" struct. Let’s see the execution.
Algorithm
Step 1 − Create a package main and declare fmt(format package) package in the program where main produces executable Example:s and fmt helps in formatting input and output.
Step 2 − Establish an interface Area of a method-signed shape ()
Step 3 − Create Rectangle struct with two fields—width and height—and a method should be used to implement the interface. Area(), which determines a rectangle's area.
Step 4 − Add a new structure of square to embed Rectangle in it.
Step 5 − Implement the Square struct's Area() method, which uses Rectangle to calculate the square's area.
Step 6 − Create a pointer to a Square struct in the main method and fill its fields with values.
Step 7 − Call the Area() function on the Square struct reference to invoke the Area method of the Square struct and print the square's area.
Step 8 − Call the Area() function on the Rectangle struct pointer as well, which prints the area of the rectangle and invokes the Rectangle struct's Area method.
Step 9 − Comparing the output of the two functions will show the difference and it will be printed in the screen using fmt.Println() function where ln means new line.
Example
In this example, we are going to using rectangle struct and square struct to show use of super keyword in class in Golang.
package main import "fmt" type Shape interface { Area() float64 //create area method in the shape interface } type Rectangle struct { width, height float64 //create width and height in the rectangle struct } func (rect *Rectangle) Area() float64 { return rect.width * rect.height //return area of rectangle } type Square struct { Rectangle } func (sq *Square) Area() float64 { return sq.Rectangle.Area() + (sq.width * 2) } func main() { sq := &Square{Rectangle{width: 10, height: 6}} fmt.Println("Area of Square:", sq.Area()) // calls the Square struct's Area method fmt.Println("Area of Rectangle:", sq.Rectangle.Area()) // calls the Rectangle struct's Area method }
Output
Area of Square: 80 Area of Rectangle: 60
Conclusion
We executed the program of using super keyword in class using two different examples. In the first example we used child struct and parent struct and in the second example we used shape interface and implemented it using rectangle and square struct.