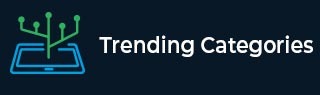
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Golang program to replace the spaces of string with a specific character
In the Go programming language, strings are a built-in data type that represents sequences of characters. They are defined using double quotes (") and can contain any valid Unicode characters The first and foremost approach used here is Replace() method which replaces the space with a specific character and many more methods can be applied to solve the problem. The modifies string will be the output printed on the console using fmt package.
Method 1: Using strings.Replace() function
In this method, the space character (" ") is replaced with the character "-" using the Replace() function. The function is instructed to replace all occurrences by the final input, "-1." Instead, you can enter a specific number if you simply want to replace a specific number of occurrences.
Syntax
strings.Replace()
The Replace() function in Golang is used to replace any instances of a particular substring in a string with another substring. The original string, the substring to be changed, and the replacement substring are the three inputs required by the function. It gives back a new string that has the requested substitutions applied.
Algorithm
Step 1 − Create a package main and declare fmt(format package) and strings package in the program where main produces executable Examples and fmt helps in formatting input and output.
Step 2 − Create an initial_string whose spaces will be replaced with a specific character.
Step 3 − Print the string on the console using print statement in Golang.
Step 4 − Use strings.Replace() function to replace the space with a new character.
Step 5 − Print the modified string on the console using fmt.Println() function where ln means new line.
Example
In the following example we are going to use the internal string function called as string.Replace() to replace the spaces of string with a specific character
package main import ( "fmt" "strings" //import fmt and strings package ) //create main function to execute the program func main() { initial_string := "Hello alexa!" //create string fmt.Println("The initial string created here is:", initial_string) replaced_string := strings.Replace(initial_string, " ", "-", -1) //replace the space in string using Replace() function fmt.Println("The replaced string after using replace method:") fmt.Println(replaced_string) //print the modified string on the console }
Output
The initial string created here is: Hello alexa! The replaced string after using replace method: Hello-alexa!
Method 2: Using a for loop
In this example we will learn how to replace the spaces of string with a specific character. Here, this approach checks to see if the current character is a space as it iterates over each character in the original string using a for-loop. If so, the replaced character is added to the string in question. If not, the character is added to the altered string in its original form. Running this program would provide the same result as the previous example, which is the phrase "Hello-alexa!"
Algorithm
Step 1 − Create a package main and declare fmt(format package) and strings package in the program where main produces executable Examples and fmt helps in formatting input and output.
Step 2 − Create a main function and in that function make a variable to hold the starting string you want to change.
Step 3 − Create a blank string variable and initialize it to hold the updated string.
Step 4 − Go through each character in the starting string one more time.
Step 5 − Add the designated replacement character to the changed string if the current character is a space.
Step 6 − Add the character to the changed string if the current character is not a space.
Step 7 − The original string should be iterated over until all characters have been handled. The changed string now includes the original string with the requested character in place of the spaces.
Step 8 − The altered string is printed on the console using fmt.Println() function where ln means new line.
Example
In the following example, we are going to use a use-defined function to replace the spaces of string with a specific character in golang
package main import ( "fmt" //import fmt package in the program ) //create main function to execute the program func main() { initial_string := "Hello alexa!" //create a string fmt.Println("The initial string created here is:", initial_string) var replaced_string string for _, ch := range initial_string { if ch == ' ' { replaced_string += "-" //if the character is empty replace it with the specified character } else { replaced_string += string(ch) //else use the same character } } fmt.Println("The replaced string given here is:") fmt.Println(replaced_string) //print the modified string using print statement in Golang }
Output
The initial string created here is: Hello alexa! The replaced string given here is: Hello-alexa!
Conclusion
We executed the program of to replacing the spaces of string with a specific character using two examples. In the first example we used the built-in Replace() function whereas in the second example we used for loop to execute the program