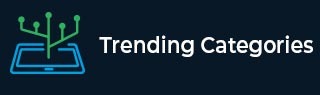
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Golang Program to Print Spiral Pattern of Numbers
In this tutorial, we will learn how to print spiral pattern of Numbers using Go programming language.
A Spiral pattern of numbers is used to print numbers in spiral pattern on the screen. In this program we will create an array of size n, store numbers in it and use the array to create a matrix in a spiral format.
Syntax
for initialization; condition; update { statement(s) } func make([]T, len, cap) []T
make() function is used to allocate memory of heap for some variables. For eg we can use make() function to allocate memory for an array. This function takes three arguments one is the data type of the array, another is the length of the array and capacity. This function returns the final array which we can store in an variable.
Example: Golang program code to print spiral pattern of numbers using for loop
Algorithm
STEP 1 − Import the package fmt
STEP 2 − Create a function name spiral() which contain the logic.
STEP 3 − Initialize the variables left, right, top, bottom and assign values to them.
STEP 4 − Use the make() function to append an array variable of integer data type and store resultant array in another variable. Decide the size of array and pass it as the argument to the array variable.
STEP 5 − Use the for loop on all the variables to arange them in a spiral format.
STEP 6 − Ararnge the central element in the spiral.
STEP 7 − Start function main()
STEP 8 − Initialize a variable named num, length and assign an array of such length.
STEP 9 − Call the spiral() function, inside a loop to print the pattern.
STEP 10 − EXIT the program.
Example
// GOLANG PROGRAM TO PRINT SPIRAL PATTERN OF NUMBERS USING FOR LOOPS. package main // fmt package have the required packages import ( "fmt" ) // CREATE A SPIRAL FUNCTION WHICH RETURNS THE REQUIRED ARRAY func spiral(n int) []int { // INITIALIZE THE REQUIRED VARIABLES left, top, right, bottom := 0, 0, n-1, n-1 // Size of the matrix array_size := n * n spiral_array := make([]int, array_size) i := 0 // USE FOR LOOP ON THE VARIABLES FOR PRINTING THE REQUIRED PATTERN for left < right { for c := left; c <= right; c++ { spiral_array[top*n+c] = i i++ } top++ for r := top; r <= bottom; r++ { spiral_array[r*n+right] = i i++ } right-- if top == bottom { break } for c := right; c >= left; c-- { spiral_array[bottom*n+c] = i i++ } bottom-- for r := bottom; r >= top; r-- { spiral_array[r*n+left] = i i++ } left++ } // center (last) element spiral_array[top*n+left] = i //RETURN THE ARRAY return spiral_array } // start the function main() // This function is the entry point of the executable program func main() { // THE VALUE TO BE PASSED IN THE spiral() Function num := 5 len := 2 fmt.Println("IT IS A SPIRAL PATTERN OF LENGTH ", num) //todraw the pattern use the for loop on spiral function for i, draw := range spiral(num) { fmt.Printf("%*d ", len, draw) if i%num == num-1 { fmt.Println("") } } }
Output
IT IS A SPIRAL PATTERN OF LENGTH 5 0 1 2 3 4 15 16 17 18 5 14 23 24 19 6 13 22 21 20 7 12 11 10 9 8
Description of Code
Declare the package main in the go lang program
We imported the fmt package that includes the files of package fmt. fmt package allows us to print anything on the screen.
Now we have created the spiral() function, and initialize the variables, Also initialize the size of the matrix to print in the spiral format by using the elements of the array.
We have created an array whose elements we will print in the spiral format using for loops.
Use the for loop to print numbers, in a spiral format.
The spiral() function, returns an array.
Start the main() function. Call the spiral function from a loop to print the elements stored in the array.
And finally printing the result in the form of a spiral pattern of numbers, using fmt.Println () function.
Conclusion
We have successfully compiled and executed a go language program to print a spiral pattern of numbers on the screen.