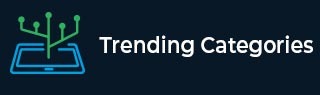
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Golang Program to print all integers between a range that aren't divisible by either 2 or 3
Let's assume the range is from 0 to 50. We have to print all the integers that aren't divisible by either 2 or 3.
Steps
- Use a for loop ranging from 0 to 50.
- Then, use an if statement to check if the number isn't divisible by both 2 and 3.
- Print the numbers satisfying the condition.
Explanation
- The for loop ranges from 0 to 50.
- The number is divided by 2 and 3.
- If the remainder ≠ 0, the number isn't divisible by either 2 and 3.
- The number satisfying the condition is printed.
Example
package main import "fmt" func main(){ for i:=1; i<=50; i++{ if !(i % 2 == 0 || i % 3 == 0){ fmt.Println(i) } } }
Output
1 5 7 11 13 17 19 23 25 29 31 35 37 41 43 47 49
Advertisements