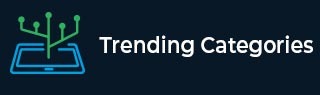
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Golang program to implement select statement
The Golang Select statement is similar to the switch statement, the switch statement selects the Output based on the cases but here the Output is selected based on which communication occurs fast in the channels.
Syntax
func make ([] type, size, capacity)
The make function in go language is used to create an array/map it accepts the type of variable to be created, its size and capacity as arguments.
time.Sleep()
This function belongs to the time package. Here, the word sleep depicts the meaning of the function which says that it will block the execution of goroutine for a specific amount of time.
time.Second()
This function is a part of the time package and it is mainly used to represent the duration of seconds.
Algorithm
Step 1 − Import the required packages in the program
Step 2 − Create a main function and in the main create two channels using built-in functions
Step 3 − Send the data to channels using go routines and use sleep to receive the message before exit of select statement
Step 4 − Use select statement to get the Output on the console from whichever channel it is received first
Step 5 − The print statement is executed using Println function from the fmt package
Example 1
In this example, we will implement select statement by creating two channels and send data to the channels using go routines. The select statements will be used to receive data from the channels and the data that is received first will be printed on the console.
package main import ( "fmt" "time" ) func main() { channel1 := make(chan string) channel2 := make(chan string) go func() { time.Sleep(2 * time.Second) channel1 <- "good" }() go func() { time.Sleep(3 * time.Second) channel2 <- "morning" }() select { case msg1 := <-channel1: fmt.Println(msg1) case msg2 := <-channel2: fmt.Println(msg2) } }
Output
good
Example 2
In this illustration, two channels will be created. The go routines will be used to send the data to the channel and then use select statement to get the Output as the data received from the channels but here a default will also be used if no message is received.
package main import ( "fmt" "time" ) func main() { channel1 := make(chan string) channel2 := make(chan string) go func() { time.Sleep(2 * time.Second) channel1 <- "good" }() go func() { time.Sleep(3 * time.Second) channel2 <- "morning" }() select { case msg1 := <-channel1: fmt.Println(msg1) case msg2 := <-channel2: fmt.Println(msg2) default: fmt.Println("No messages received") } }
Output
No messages received
Conclusion
We compiled and executed the program of implementing the select statement using two examples. In both the examples we used similar channels but the only thing that makes them different is the use of the default in the second example if no message is received from the channel.