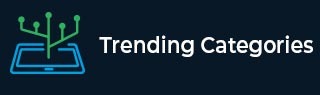
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Golang program to implement recursive anonymous function
In this Go language article, we will write programs to implement recursive anonymous function by generating fibonacci numbers, by using cache, as well as factorial of a number.
An anonymous function is the function which does not have its name and it calls itself within its own body and when repeated calls are made, it called as recursive functions.
Method 1
In this Example, we will generate Fibonacci numbers recursively by using an anonymous function. Here, fibo is assigned to the anonymous function with func and an input parameter.
Algorithm
Step 1 − Create a package main and declare fmt(format package) package in the program where main produces executable codes and fmt helps in formatting input and output.
Step 2 − Create a main function and in that function further create an anonymous function fibo with one parameter n which signifies the location of which fibonaaci number is to be calculated
Step 3 − The Algorithm is set recursively as in the base condition is checked if n is less than equal to 1 return the n
Step 4 − Follow the recursive pattern of fibo(n-1)+ fibo(n-2) and keep following until base condition is not satisfied. When the base condition is successfully executed return the values in the stack and add them as per the statement
Step 5 − Finally, the end output is generated and printed on the console using Println function from the fmt package
Example
The following is the golang program to implement recursive anonymous function by generating Fibonacci numbers
package main import "fmt" func main() { var fibo func(n int) int fibo = func(n int) int { if n <= 1 { return n } return fibo(n-1) + fibo(n-2) } fmt.Println("The fibonacci number at location 6th is:") fmt.Println(fibo(6)) // output: 8 }
Output
The fibonacci number at location 6th is: 8
Method 2
In this method, cache is used specifically to store the previous calculations of Fibonacci numbers, once the output will be received it will be stored inside the cache and returned back.
Algorithm
Step 1 − Create a package main and declare fmt(format package) package in the program where main produces executable codes and fmt helps in formatting input and output.
Step 2 − Create a closure which will return the function having input n representing the nth Fibonacci number
Step 3 − In the function create a cache using make built-in function that is used to store the previous calculations of Fibonacci numbers
Step 4 − The inner fib function is the recursive anonymous function which will be recursively executed until the base condition is satisfied
Step 5 − The output after its calculated is stored in the cache and returned to the function
Step 6 − The output is printed using Println function from the fmt package where ln means new line
Example
The following is the Golang program to implement recursive anonymous function by using cache
package main import "fmt" func main() { fibo := func() func(int) int { cache := make(map[int]int) var fib func(int) int fib = func(n int) int { if n <= 1 { return n } if val, ok := cache[n]; ok { return val } cache[n] = fib(n-1) + fib(n-2) return cache[n] } return fib }() fmt.Println("The fibonacci number at the location 6th is:") fmt.Println(fibo(6)) }
Output
The fibonacci number at the location 6th is: 8
Method 3
In this method, we will write a Go language program to implement recursive anonymous function by calculating the factorial of a number.
Algorithm
Step 1 − Create a package main and declare fmt(format package) package in the program where main produces executable codes and fmt helps in formatting input and output.
Step 2 − Create a main function in which define an anonymous function of factorial of type int
Step 3 − Run the anonymous function in that function check if the value in the input is equals to 0 return 1
Step 4 − If the value is not zero run the function recursively to find factorial of the number
Step 5 − Then, call the function with an input parameter whose factorial is to be calculated
Step 6 − Print the factorial on the console using Println function from the fmt package where ln refers to new line
Example
The following is the Golang program to implement recursive anonymous function using factorial of a number
package main import "fmt" func main() { var factorial func(int) int factorial = func(val int) int { if val == 0 { return 1 } return val * factorial(val-1) } output := factorial(6) fmt.Println("The factorial of the given number is:") fmt.Println(output) }
Output
The factorial of the given number is: 720
Conclusion
We compiled and executed the program of implementing the recursive anonymous function. In the first Example, anonymous function is used to calculate the nth fibonacci number, in the second Example, cache along with anonymous function is used and in the third Example factorial of a number is calculated recursively.