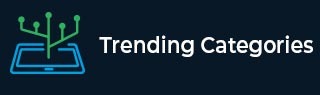
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Golang Program to Get the size of a directory
In golang, it is easy to get the size of file, but getting the size of a directory is a bit complex. Here in this article we will use os.Open() function , walk function as well as readdir() function to get the size of a directory.
Syntax
funcReadDir(dirname string) ([]fs.FileInfo, error)
The ReadDir() function is present in os package and is used to read the directory. The function accepts one argument which is the name of the directory which is to be read and returns a list FileInfo for directory’s content. If an error is generated while reading the directory then only an error variable is returned.
Example 1
In this Example we will write a go language program to get the size of a directory by using the open and stat functions present in os package. The os package provides functions for interacting with the operating system, including opening and reading files and directories.
package main import ( "fmt" "os" ) func main() { dir, err := os.Open("new") if err != nil { fmt.Println(err) return } defer dir.Close() fileInfo, err := dir.Stat() if err != nil { fmt.Println(err) return } fmt.Println("Directory size:", fileInfo.Size(), "bytes") }
Output
Directory size: 4096 bytes
Example 2
In this Example we will write a go language program to get the size of a directory by using the walk function present in the path package. The filepath package provides functions for manipulating file paths, including walking a directory tree. In this method, we will use the filepath.Walk function to recursively walk the directory tree and sum the sizes of all the files and subdirectories.
package main import ( "fmt" "os" "path/filepath" ) func main() { var totalSize int64 err := filepath.Walk("new", func(path string, info os.FileInfo, err error) error { if !info.IsDir() { totalSize += info.Size() } return nil }) if err != nil { fmt.Println(err) return } fmt.Println("Directory size:", totalSize, "bytes") }
Output
Directory size: 673 bytes
Example 3
In this Example we will write a go language program to get the size of the directory by using the readDir() function present in the ioutil package. ioutil.ReadDir function to read the contents of the directory and then sum the sizes of all the files and subdirectories.
package main import ( "fmt" "io/ioutil" ) func main() { files, err := ioutil.ReadDir("new") if err != nil { fmt.Println(err) return } var totalSize int64 for _, file := range files { if !file.IsDir() { totalSize += file.Size() } } fmt.Println("Directory size:", totalSize, "bytes") }
Output
Directory size: 673 bytes
Conclusion
We have successfully compiled and executed a go language program to get the size of a directory along with Examples. Here we have mentioned three different methods for getting the size of a directory in Golanguage. Each method has its advantages and disadvantages, and the choice of method depends on the specific requirements of the task at hand. Whichever method you choose, it's essential to consider its suitability for your specific requirements and to handle errors appropriately to ensure reliable and correct results.