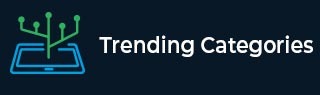
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Golang Program to get the remainder of float numbers using library function
In this article we will discuss about how to get the remainder of float numbers using library function in Go language.
In programming, a float is a number that has a decimal point in it. For example: 0.2, 5.89, 20.79, etc.
Remainder: Remainder in division is defined as the result left after the division process is over. For example, if 4 is the dividend and 2 is the divisor then after dividing 4 with 2 the remainder will be 0. Similarly on dividing 4 with 3 the remainder will be 1.
To get the remainder of floating values we can use math.Mod() library function. The Syntax of mod() function is defined below as −
func mod(x, y float64) float64.
This function takes two arguments of float data type. Here they are depicted by x and y where x is the dividend and y is the divisor. This function returns the remainder as a float number.
The source code to get the remainder of float numbers using library function is compiled and executed below.
Algorithm
Step 1 − Import the package fmt and math.
Step 2 − Start the main() function.
Step 3 − Initialize the variables of data type float and assign values to them.
Step 4 − Implement the logic.
Step 5 − Print the result on the screen.
Example
package main import ( "fmt" "math" ) // fmt package allows us to print anything on the screen. // math package allows us to use predefined mathematical methods like mod(). // calling the main() function func main() { // initializing the variables to store the dividend, divisor and result respectively. var dividend, divisor, result float64 // assigning values of the dividend and the divisor dividend = 36.5 divisor = 3 // performing the division and storing the results result = math.Mod(dividend, divisor) // printing the results on the screen fmt.Println("The remainder of ", dividend, "/", divisor, "is: ") // limiting the result upto two decimal points fmt.Printf("%.2f", result) }
Output
The remainder of 36.5 / 3 is: 0.50
Description of the Code
First, we to import the fmt package and math package respectively that allows us to print anything on the screen and use mathematical methods respectively.
Then we call the main() function.
After that we have to initialize 64-bit variables of float data type to assign the value of dividend, devisor and to store the results.
Note that we have initialized 64 bit variables because mod() function accepts 64 bit numbers as arguments.
Call Mod() method defined in math package and pass the value of dividend and divisor as arguments in it.
Store the value returned by the function in a separate variable created above as result.
Print the final results on the screen by using fmt.Println() function.
Algorithm
Step 1 − Import the package fmt and math.
Step 2 − Initialize and define the getRemainder() function.
Step 3 − start the main() function.
Step 4 − Initialize the variables of data type float and assign the value of dividend and divisor in them.
Step 5 − Call the getRemainder() function.
Step 6 − Store its results in a variable.
Step 7 − Print the results on the screen.
Example
The source code to get the remainder of a float number using this approach is compiled and executed below.
package main import ( "fmt" "math" ) // fmt package allows us to print anything on the screen. // math package allows us to use other pre-defined mathematical methods like mod(). // creating and defining the getRemainder() function. func getRemainder(dividend, divisor float64) float64 { // initializing a 64 bit value variable to store the result of division process var value float64 // performing the division and storing the results value = math.Mod(dividend, divisor) // returning the value of result return value } // calling the main() function func main() { // initializing the variables to store the dividend, divisor and result respectively. var dividend, divisor, result float64 // storing the values of dividend and divisor in the variables dividend = 20.5 divisor = 5 // storing the result result = getRemainder(dividend, divisor) // printing the results on the screen fmt.Println("The remainder of ", dividend, "/", divisor, "is: ") // limiting the result upto two decimal points fmt.Printf("%.2f", result) }
Output
The remainder of 20.5 / 5 is: 0.50
Description of the Code
First, we have to import the fmt package and math package respectively that allows us to print anything on the screen and use mathematical methods respectively.
Then we have created and defined the getRemainder() function that will take care of the logic and will return the final value after doing all the operation.
After that we have to initialize and assign the value of dividend and divisor.
Note that we have initialized 64 bit variables because mod() function accepts 64 bit numbers as arguments.
Then we have to call the getRemainder() function and pass the values of dividend and divisor in them as arguments.
In getRemainder() function we have used math.Mod() method to implement the logic.
Return the value of the result of division which in this case is the remainder.
Store the value returned by the function in a separate variable. We have named it as result.
Print the results on the screen by using fmt.Println() function.
Conclusion
We have successfully compiled and executed the Go language program to get the remainder of float numbers using library functions along with examples.