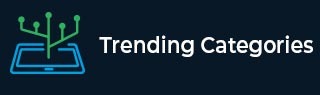
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Golang program to find the prime numbers from the array
In this tutorial, we will showcase how to find the prime numbers from the array using different examples. Prime numbers are those numbers which are either divisible by 1 or by themselves they have no factor other than this. Now we will present you few illustrations to help you get clear with the logic behind this program.
Method 1: Finding Prime Number in the main Function
In this particular method, we will print the prime numbers with the help of nested for loops. We will half the array elements in every inner iteration and check whether they are divisible or not by other numbers. Let’s have a look and comprehend it.
Algorithm
Step 1 − Create a package main and import fmt package in the program.
Step 2 − Create a main function to execute the program and further create an array inside the main function from which the prime numbers have to be searched.
Step 3 − Create a variable named flag inside the main with initial value assigned as 0.
Step 4 − Run a loop from i=2 till i<=len(arr)-1 where this loop is an outer loop and j=2 till j<arr[i]/2 is an inner loop, the inner loop will execute with every iteration of outer loop.
Step 5 − Initialize the flag=0 when the outer loop begins and in the first iteration of inner loop check whether arr[i]%j==0.
Step 6 − If the condition is satisfied set the flag equals to 1 and break the loop because if the element is not divisible till its half it will not be divisible ahead by any other number.
Step 7 − Run the loop till it terminates and if the condition is not satisfied in any iteration, outside the loop check a condition if flag is equal to 0 or the array element is greater than 1.
Step 8 − If this condition is found to be true , this means that the element is a prime number and hence print it.
Step 9 − The print statement is executed using fmt.printf() function with %d inside it that is used to print the array element.
Example
Golang program to find the prime numbers by doing half of elements of the array in the main function
package main import "fmt" func main() { arr := [6]int{10, 11, 12, 13, 65, 73} fmt.Println("The array elements are: ", arr) var flag int = 0 fmt.Printf("Prime Numbers: \n") for i := 0; i <= len(arr)-1; i++ { flag = 0 for j := 2; j < arr[i]/2; j++ { if arr[i]%j == 0 { flag = 1 break } } if flag == 0 && arr[i] > 1 { fmt.Printf("%d ", arr[i]) } } }
Output
The array elements are: [10 11 12 13 65 73] Prime Numbers: 11 13 73
Method 2: Using Square root in User-Defined Function
In this method,we will create an external function with the range as parameter and further a sqrt function will be used to find the square root of elements from which we can check whether the number is divisible up to that range or not. Let’s have a look to know more about it.
Algorithm
Step 1 − Create a package main and import fmt and math package in the program.
Step 2 − Create a function named printPrimeNumbers with parameters num1 and num2 signifying the range from which prime numbers are to be searched.
Step 3 − In the function check the condition that is the range num1 is less than 2 and also num is also less than 2 , is the condition is satisfied, print numbers are greater than 2 and return.
Step 4 − Run a loop that until num1<=num2 set isprime flag equals to true and run an inner loop from i=0 till i <= int(math.Sqrt(float64(num1)) where sqrt is used to find square root of the array element.
Step 5 − Check the condition that if the num1is divisible set isprime as false, break the loop and increase the value of num1.
Step 6 − but if the condition is not satisfied in any iteration the loop terminates and prime number is printed.
Step 7 − Call the function from the main with the range elements as arguments.
Step 8 − The print statement is executed using fmt.Println() function.
Example
Golang program to find the prime numbers from an array using sqrt function in the user-made function
package main import ( "fmt" "math" ) func printPrimeNumbers(num1, num2 int) { if num1 < 2 || num2 < 2 { fmt.Println("Numbers must be greater than 2.") return } for num1 <= num2 { isPrime := true for i := 2; i <= int(math.Sqrt(float64(num1))); i++ { if num1%i == 0 { isPrime = false break } } if isPrime { fmt.Printf("%d ", num1) } num1++ } } func main() { var range1 int = 4 var range2 int = 100 fmt.Println("The range from which prime numbers have to be searched is from", range1, "to", range2) fmt.Println("The following prime numbers are: ") printPrimeNumbers(4, 100) }
Output
The range from which prime numbers have to be searched is from 4 to 100 The following prime numbers are: 5 7 11 13 17 19 23 29 31 37 41 43 47 53 59 61 67 71 73 79 83 89 97
Conclusion
In the above tutorial, we searched for prime numbers in the array using two methods. In the first method we used the main function in which we halved the array element and searched for prime numbers. In the second method we used an external function with sqrt method to find the square root of array elements and print the prime numbers. Hence, all the methods executed successfully.