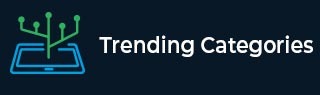
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Golang program to find if a given subarray exists in a given array using two pointer approach
In this Golang article, we are going to find if a given subarray exists in a given array using two pointer approach with iterative and optimized-iterative method. An array is a collection of elements of the same data type, arranged in a contiguous block of memory, and accessed using an index or a subscript.
Syntax
func isSubarrayPresent(arr []int, sub []int) bool {…}
The isSubarrayPresent() function is used to find if a given subarray exists in a given array using two pointer approach. It takes array and sub-array as its argument.
Algorithm
Step 1 − First, we need to import the fmt package.
Step 2 − Now, create an isSubarrayPresent() function that takes an array and a subarray of type integer. This function will return a boolean value indicating if sub-array is present in array or not.
Step 3 −
For example 1 −
If the length of the sub-array is zero that means it is empty and present in any array. So, true is returned in such case. Otherwise, use for loop to match the elements of the sub-array with the array using certain conditions. And if all the elements of the sub-array are present in array then, return true else false.
For example 2 −
It first checks if the length of sub-array is greater than the length of array, and if it so then it returns false. Otherwise, use for loop with if-else conditions to match the elements of the sub-array with the array using certain conditions. If there is a match, the function increments j to move on to the next element of sub-array. If there is no match, the function resets j to zero and increments i to move on to the next element of array. And if all the elements of the sub-array are present in array then, return true else false
Step 4 − Start the main() function. Inside the main() function, initialize the array and sub-arrays.
Step 5 − Now, calls the isSubarrayPresent() function and pass the array and sub-arrays to it.
Step 6 − Further, the resultant boolean value is printed using the fmt.Println() function.
Example 1
In this example, we will define a isSubarrayPresent() function using iterative approach that is used to find if a given subarray exists in a given array using two pointer approach.
package main import "fmt" func isSubarrayPresent(arr []int, sub []int) bool { if len(sub) == 0 { return true } i := 0 j := 0 for i < len(arr) { if arr[i] == sub[j] { j++ if j == len(sub) { return true } } else { i -= j j = 0 } i++ } return false } func main() { arr := []int{1, 2, 3, 4, 5, 6, 7, 8, 9} sub1 := []int{2, 3, 4} sub2 := []int{4, 3, 2} sub3 := []int{1, 2, 3, 4, 5, 6, 7, 8, 9, 10} fmt.Println(isSubarrayPresent(arr, sub1)) fmt.Println(isSubarrayPresent(arr, sub2)) fmt.Println(isSubarrayPresent(arr, sub3)) }
Output
true false false
Example 2
In this example, we will define a isSubarrayPresent() function using iterative approach in optimized manner that is used to find if a given subarray exists in a given array using two pointer approach.
package main import "fmt" func isSubarrayPresent(arr []int, sub []int) bool { n := len(arr) m := len(sub) if m > n { return false } i := 0 j := 0 for i < n && j < m { if arr[i] == sub[j] { j++ } else if j > 0 && arr[i] == sub[j-1] { j-- continue } else { i = i - j j = 0 } i++ } return j == m } func main() { arr := []int{10, 20, 30, 40, 50, 60, 70, 80, 90} s1 := []int{40, 50, 9} s2 := []int{5, 2, 10} s3 := []int{10, 20, 30, 40, 50} fmt.Println(isSubarrayPresent(arr, s1)) fmt.Println(isSubarrayPresent(arr, s2)) fmt.Println(isSubarrayPresent(arr, s3)) }
Output
false false true
Conclusion
We have successfully compiled and executed a go language program to find if a given subarray exists in a given array using two pointer approach along with two examples. In the first example, we have used the iterative method and in the second example, we have used the optimized-iterative method.