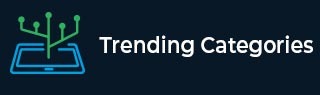
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Golang Program to convert long type variables to int
In go language, a long is not a separate data type but it is an extension made from the integer data type to store even larger values of integers. The main difference between an int data type and long data type is that an int data type is 32 bits whereas long data type is 64 bits. Here we will learn about different techniques to convert long types variables to int using go programming language.
Method 1: By Using Type Casting
In this example we will use the type casting approach to convert the long data type variables to int. Type casting works by passing the variable as an argument to int() function.
Syntax
func typeOf (x interface{})
The typeOf() function is used to get the type of any variable. This function is present in reflect package and it takes the variable whose type is to be determined as an argument. The function then returns the type of the variable specified as the result.
Algorithm
Step 1 − First, we need to import the fmt and reflect packages.
Step 2 − Then, start the main() function.
Step 3 − Inside the main initialize the variable of long data type named x and store value to it.
Step 4 − Print the variable on the screen using fmt.Println() function.
Step 5 − Print the type of this variable by using TypeOf() function present in reflect package.
Step 6 − Store the result in a new variable of same data type.
Step 7 − Print the data inside this variable on the screen along with its type using TypeOf() function.
Example
Go language program to convert long type variable to int by using type casting
package main import ( "fmt" "reflect" ) func main() { var x int64 = 5 fmt.Println("The variable to be converted is:", x) fmt.Println("Type of x is:", reflect.TypeOf(x)) fmt.Println() var y int = int(x) fmt.Println("The variable obtained after converting the above value to int is:", y) fmt.Println("Type of y is:", reflect.TypeOf(y)) }
Output
The variable to be converted is: 5 Type of x is: int64 The variable obtained after converting the above value to int is: 5 Type of y is: int
Method 2: Using And Operator
In this method, we will use and (&&) operator in order to convert a long type variable to int by using and operator. The && operator is used to remove the extra bits from the long data type variable.
Algorithm
Step 1 − First, we need to import the fmt package.
Step 2 − Then start the main() function and initialize a variable of long type and assign value to it.
Step 3 − Print the variable on the screen and use the & operator in order to remove the extra bits in the long type.
Step 4 − print the result on the screen along with its type.
Example
Go language program to convert long type variable to int by using and operator
package main import ( "fmt" "reflect" ) func main() { var x int64 = 51 fmt.Println("The variable to be converted is:", x) fmt.Println("Type of x is:", reflect.TypeOf(x)) fmt.Println() var y int = int(x & 0x7fffffff) fmt.Println("The variable obtained after converting the above value to int is:", y) fmt.Println("Type of y is:", reflect.TypeOf(y)) }
Output
The variable to be converted is: 51 Type of x is: int64 The variable obtained after converting the above value to int is: 51 Type of y is: int
Conclusion
We have successfully compiled and executed a go language program to convert a long type variable to int along with examples. We have used two programs here the first program uses the type casting approach in which we are simply passing the variable to be converted as argument in the int() function while in the second program we have used AND operator in order to remove the extra bits of long data type variable.