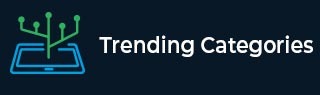
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Golang Program to Convert List to Set
In this tutorial, we will write a golang program to convert a list to set. A linked list is a structure that is created dynamically it has two elements one to store the value and other to store the address of the next structure. A set is an abstract data type that can store specific values without repeating any of them and without any particular sequence.
Convert a Linked List to a Set
Now let us look at an example to convert the linked list to a set.
Syntax
func make([]type, length, capacity) []type
The make function is used to make a slice or map. It takes three arguments one is the slice name and type that we wish to make followed by the length and capacity of the slice. The function then returns the final slice.
Algorithm
Step 1 − First, we need to import the fmt package.
Step 2 − The next step is to create a node. For that we are defining a new structure called node.
Step 3 − Then we need to create a linkedlist struct. It also has two fields one is the pointer variable called head. This variable points to the head node
Step 4 − Then we have initialized two functions one is the initlist() function. This function is defined on the linkedlist struct and returns the address of the linkedlist{}.
Step 5 − The second function is named as prepend and it is used to add a new node element to the linked list and takes a node as an argument.
Step 6 − Once the data is added to the current node of linked list we need to make the next pointer of linked list to point to the next node and increment the length of linked list
Step 7 − Start the main function and create a new linked list named mylist by calling the initlist() function.
Step 8 − After that we have created couple of nodes and stored string values to them.
Step 9 − The next step is to arrange these nodes together to form a linked list for that we are calling prepend() function by passing each node as an argument to the function.
Step 10 − Then we need to create a set. We have named it as newset and it stores data in string format.
Step 11 − The next step is to iterate over the linked list and on each iteration we are extracting value from the linked list and are storing it on the set.
Step 12 − Then we need to make the pointer variable of linked list to point to the next node and repeat this process until we get the nil or null value.
Step 13 − The next step is to print the set for that we are using fmt.Println() function.
Example
package main import ( "fmt" ) type node struct { data string next *node } type linkedlist struct { len int head *node } func initList() *linkedlist { return &linkedlist{} } func (l *linkedlist) prepend(n *node) { node := &node{ data: n.data, } if l.head == nil { l.head = node } else { node.next = l.head l.head = node } l.len++ return } func main() { mylist := initList() node1 := &node{data: "Apple"} node2 := &node{data: "mango"} node3 := &node{data: "Banana"} mylist.prepend(node1) mylist.prepend(node2) mylist.prepend(node3) newset := make(map[string]struct{}) for mylist.head != nil { newset[mylist.head.data] = struct{}{} mylist.head = mylist.head.next } fmt.Println("The obtained set is:", newset) }
Output
The obtained set is: map[Banana:{} mango:{} Apple:{}]
Conclusion
We have successfully compiled and executed a go language program to covert a linked list to set along with an example.