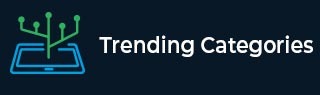
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Golang Program to check the given number is an odd number using library function
In this article we will discuss about how to check if a given number is odd using library function Go language.
ODD NUMBERS − In mathematics any number is called odd if it gives “1” as remainder when divided by “2”. For example 1, 3, 5, 7 ….
Even Numbers − unlike odd numbers even numbers get completely divided by “2” and do not produce any remainder. For example − 2, 4, 6, 8 …
Syntax
func Mod(number1, number2 float64) float64
The mod() function is defined in the math package. This function returns the remainder by dividing the two numbers passed to it as arguments. It accepts arguments as 64-bit float numbers and returns the result as a 64-bit float number.
The source code to check if a given number is odd is compiled and executed below.
Example 1: Golang Program to check the Given Number is an Odd Number Using Library Function
Algorithm
Step 1 − Import the package fmt.
Step 2 − start the main function().
Step 3 − Initialize a variable of data type float and store value in it.
Step 4 − Apply the logic.
Step 5 − print the results on the screen.
Example
// Golang Program to check the given number is an odd number using library function package main import ( "fmt" "math" ) // fmt package provides the function to print anything // math package allows us to use other predefined mathematical methods. func main() { // initializing a number variable and assigning value to it. var number float64 // assigning value to it. number = 1009 // initializing the result variable var result float64 // dividing the said number by 2 and storing the result in a variable result = math.Mod(number, 2) // Implementing the logic to get the odd number if result != 0 { fmt.Println("THE NUMBER", number, "IS A ODD NUMBER") } else { fmt.Println("THE NUMBER", number, "IS NOT AN ODD NUMBER") } }
Output
THE NUMBER 1009 IS A ODD NUMBER
Description of the Code
1. First, we import the package fmt that allows us to print anything and math that allows us to use other predefined mathematical methods.
2. Then we start the main() function.
3. Inititalize and assign value to a 64-bit float type variable. We have chosen float here because math.Mod() accepts a 64-bit float as input value.
4. Use the math.Mod() to divide the number by 2 and store the remainder in a separate 64 bit float type variable.
5. Use the if condition to check if the remainder obtained above is zero or not.
6. If it is not zero then print that the number is odd number otherwise print on the screen that the number is even.
Example 2: The Following is an Example to check if the Given Number is an Odd Number using Two Different Functions.
Algorithm
Step 1 − Import the package fmt.
Step 2 − Initialize and define the getOdd() function.
Step 3 − start the main function().
Step 4 − Initialize a variable of data type float and store value in it.
Step 5 − Call the getOdd() function.
Step 6 − print the results on the screen
Example
// Golang Program to check the given number is an odd number using library function package main import ( "fmt" "math" ) // fmt package provides the function to print anything // math package allows us to use other predefined mathematical methods. // Defining getOdd() function func getOdd(num float64) { // initializing the result variable var result float64 // dividing the said number by 2 and storing the result in a variable result = math.Mod(num, 2) // Implementing the logic to get the odd number if result != 0 { fmt.Println("THE NUMBER", num, "IS A ODD NUMBER") } else { fmt.Println("THE NUMBER", num, "IS NOT AN ODD NUMBER") } } func main() { // calling findEven function and print the result getOdd(1) fmt.Printf("\n") // printing a new line getOdd(99) fmt.Printf("\n") getOdd(87) fmt.Printf("\n") getOdd(20) }
Output
THE NUMBER 1 IS A ODD NUMBER THE NUMBER 99 IS A ODD NUMBER THE NUMBER 87 IS A ODD NUMBER THE NUMBER 20 IS NOT AN ODD NUMBER
Description of the Code
1. First, we import the package fmt that allows us to print anything and math that allows us to use other predefined mathematical methods.
2. Then we have initialized and defined the getOdd() function that will take care of our logic.
3. Then we start the main() function.
4. Call the getOdd() function by passing the number as arguments to it.
5. The getOdd() function is accepting input in 64-bit float data type format because Mod() method accepts argument in float format.
6. Use the math.Mod() to divide the number by 2 and store the remainder in a separate 64 bit float type variable.
7. Use the if condition to check if the remainder obtained is zero or not.
8. If it is not zero then print that the number is odd number otherwise print on the screen that the number is even.
Conclusion
We have successfully compiled and executed the Go language program that will tell whether the number is odd or not along with examples using library functions.