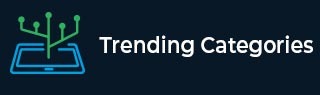
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Golang Program to Check if a string is a valid shuffle of two distinct strings
In the Go programming language, strings are a built-in data type that represents sequences of characters. They are defined using double quotes (") and can contain any valid Unicode characters. In this article, we will learn how to check if a string is a valid shuffle of two strings using different set of examples. The output will be a Boolean value printed on the console using fmt package.
Method: Using a for loop with len method
In this method, we will learn how to check if a string is valid shuffle of two distinct strings. This program defines a function called isShuffle(str1, str2, shuffle string) bool that accepts three strings as arguments: str1 and str2 are the two separate original strings, and shuffle is the string being checked to see if it was successfully shuffled with str1 and str2.
Algorithm
Step 1 − Create a package main and declare fmt(format package) package in the program where main produces executable Examples and fmt helps in formatting input and output.
Step 2 − Create a function isShuffle and in that function take mystr1, mystr2 and shuffle string as inputs.
Step 3 − The i j, and k should all be initialized to 0.
Step 4 − Repeat the shuffle string's length times k times, starting at 0.
Step 5 − Check each time to see if i is less than mystr1's length and if mystr1's i-th character corresponds to the shuffle string's k-th character. In that case, raise i.
Step 6 − If the aforementioned condition is false, see if j is smaller than mystr2's length and if mystr2's jth character is the same as the shuffle string's kth character. In that case, raise j.
Step 7 − Return false if none of the aforementioned statements is accurate and return true once the loop has finished.
Step 8 − By comparing the characters of the shuffle string with the characters of the original strings, the aforementioned method employs a single pointer approach (i,j,k) to determine whether the shuffle string is a legitimate shuffle of two separate strings.
Example
In this example, we are going to use a for loop with len function to Check if a string is a valid shuffle of two distinct strings in golang
package main import "fmt" func isShuffle(mystr1, mystr2, shuffle string) bool { if len(mystr1)+len(mystr2) != len(shuffle) { return false } i, j, k := 0, 0, 0 for k < len(shuffle) { if i < len(mystr1) && mystr1[i] == shuffle[k] { i++ } else if j < len(mystr2) && mystr2[j] == shuffle[k] { j++ } else { return false } k++ } return true } func main() { mystr1 := "abc" //create a string1 fmt.Println("The string1 given here is:", mystr1) mystr2 := "def" //create string2 fmt.Println("The string2 given here is:", mystr2) fmt.Println("Is the string a valid shuffle of two strings?") shuffle := "abcdef" fmt.Println(isShuffle(mystr1, mystr2, shuffle)) //check if the shuffle is valid }
Output
The string1 given here is: abc The string2 given here is: def Is the string a valid shuffle of two strings? true
Method 2: Using rune method
In this method, we will check if a string is a valid shuffle of two distinct strings using a for loop. The isShuffle function, which this program defines, accepts three strings: mystr1, mystr2, and shuffle. The function loops through each character in the shuffle string using two pointers, one for each input string. The function examines whether the current character and the character at the current pointer for mystr1 or mystr2 for each character in the shuffle string.
Algorithm
Step 1 − Create a package main and declare fmt(format package) package in the program where main produces executable Examples and fmt helps in formatting input and output.
Step 2 − To point to the beginning of the input strings mystr1 and mystr2, respectively, initialize two pointers, i and j.
Step 3 − Repeat after every character in the shuffled string. Check whether the current character and the character at the current pointer for mystr1 or mystr2 for each character in the shuffle string.
Step 4 − Increase the pointer for mystr1 if the current character matches the character at the current pointer for mystr1.
Step 5 − Increase the pointer for mystr2 if the current character matches the character at the current pointer for mystr2.
Step 6 − Return false if the current character for either input string does not match the character at the current pointer (indicating that the shuffle string is not a valid shuffle of the two input strings).
Step 7 − Return true if both of the input strings' pointers have reached the end of their respective strings (indicating that the shuffle string is a valid shuffle of the two input strings).
Step 8 − The output will be a Boolean value printed on the console using fmt.Println() function where ln means new line.
Example
In this example, we are going to use rune method to check if a string is a valid shuffle of two distinct strings in golang
package main import "fmt" func isShuffle(mystr1, mystr2, shuffle_val string) bool { i, j := 0, 0 // Iterate over each character in the shuffle string for _, c := range shuffle_val { if i < len(mystr1) && c == rune(mystr1[i]) { i++ } else if j < len(mystr2) && c == rune(mystr2[j]) { j++ } else { return false } } return i == len(mystr1) && j == len(mystr2) } func main() { // Test cases fmt.Println("Whether the string is a valid shuffle of two distinct strings?") fmt.Println(isShuffle("abc", "def", "dabecf")) // true }
Output
Whether the string is a valid shuffle of two distinct strings? true
Conclusion
We executed the program of checking if a string is a valid shuffle of two strings using two examples. In the first example we used for loop with len method and in the second example we used rune method.