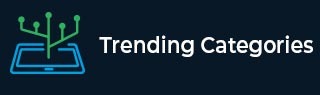
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Golang program to calculate union of two slices
In golang, a slice is known as dynamic array where it's value is not fixed and can be changed. It is more efficient and faster compared to simple arrays. In this article, we are going to learn how to calculate a union of two different slices using examples.
Syntax
func make ([] type, size, capacity)
The make function in go language is used to create an array/map it accepts the type of variable to be created, its size and capacity as arguments
func append(slice, element_1, element_2…, element_N) []T
The append function is used to add values to an array slice. It takes number of arguments. The first argument is the array to which we wish to add the values followed by the values to add. The function then returns the final slice of array containing all the values.
Algorithm
Step 1 − Create a package main and declare fmt(format package) package in the program where main produces executable codes and fmt helps in formatting input and output.
Step 2 − Create a main function and in that function create a slice1 and slice2 and print the slices on the console.
Step 3 − Call the function union_ele with slice1 and slice2 as parameters which are to be combined with each other.
Step 4 − Create an empty map which will be used to store the elements of the union using make function which is a built-in function.
Step 5 − Create a slice named output to store the union of elements using make function which is a built-in function.
Step 6 − Run a loop till the range of slice1 and check for each element if it exists in the loop don’t add it to map otherwise add it to the map and using append function add it to the output slice.
Step 7 − Similarly repeat the same process with slice2 and using append function add it to the output slice.
Step 8 − Return the output to the function and it will printed on the console using fmt.Println() function where ln means new line.
Example 1
In this example, we will learn how to calculate union of two slices using an external function. The make and append built-in functions will play a major role in this example. Let’s go through the algorithm and the code to understand the concept.
package main import ( "fmt" ) func union_ele(myslice1, myslice2 []int) []int { // Create a map to store the elements of the union values := make(map[int]bool) for _, key := range myslice1 { // for loop used in slice1 to remove duplicates from the values values[key] = true } for _, key := range myslice2 { // for loop used in slice2 to remove duplicates from the values values[key] = true } // Convert the map keys to a sliceq5 output := make([]int, 0, len(values)) //create slice output for val := range values { output = append(output, val) //append values in slice output } return output } func main() { myslice1 := []int{10, 20, 30, 40} //create slice1 fmt.Println("The elements of slice1 are:", myslice1) myslice2 := []int{30, 40, 50, 60} //create slice2 fmt.Println("The elements of slice2 are:", myslice2) fmt.Println("The union of elements presented here is:") fmt.Println(union_ele(myslice1, myslice2)) //print union of two slices }
Output
The elements of slice1 are: [10 20 30 40] The elements of slice2 are: [30 40 50 60] The union of elements presented here is: [60 10 20 30 40 50]
Example 2
In this example we will learn how we can use nested loop to calculate union of two slices with built-in functions make and append function. The output will be printed on the console. Let’s see how it is executed.
package main import ( "fmt" ) func union_ele(myslice1, myslice2 []int) []int { elements := make(map[int]bool) //create empty map output := make([]int, 0) //create output slice for i := range myslice1 { elements[myslice1[i]] = true output = append(output, myslice1[i]) } for i := range myslice2 { if _, ok := elements[myslice2[i]]; !ok { elements[myslice2[i]] = true output = append(output, myslice2[i]) } } return output //return union of two slices } func main() { myslice1 := []int{10, 20, 30, 40} //create slice1 fmt.Println("The elements of slice1 are:", myslice1) myslice2 := []int{30, 40, 50, 60} //create slice2 fmt.Println("The elements of slice2 are:", myslice2) fmt.Println("The slice after union of elements is presented as:") fmt.Println(union_ele(myslice1, myslice2)) //print union of slices }
Output
The elements of slice1 are: [10 20 30 40] The elements of slice2 are: [30 40 50 60] The slice after union of elements is presented as: [10 20 30 40 50 60]
Conclusion
In the above program, we used two examples print union of two slices. In the first example we used a for loop and in the second example we used nested for loop. Hence, program executed successfully.