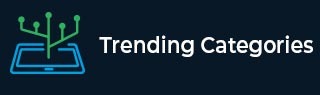
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Golang program to calculate the volume and area of a Cone
In this tutorial, we will be discussing the approach to calculate the volume and area of a cone using its height and radius in Golang programming.
But before writing the code for this, let’s briefly discuss the cone and its volume and area.
Cone
A cone is a three-dimensional figure that is pointed at the top and its base is flat and curved. The height of the cone is denoted by ‘h’ and the radius of the flat curved surface is denoted by ‘r’. An ice cream cone is a good example of a cone.
$$\mathrm{where\: length \, =\, \sqrt{(r^{2} + h^{2}))}}$$
Volume of a cone
The capacity or amount of space a cone occupies is termed its volume. Calculating the volume of a cone can be beneficial in situations where we want to know how much ice cream we can fill in a cone.
$$\mathrm{Volume = (1/3) \ast \pi \ast (r)^{2} \ast h}$$
Area of a cone
The total area occupied by the cone is known as the surface area of the cone.
$$\mathrm{Area \, =\, \pi \ast r \ast (r+l) }$$
$$\mathrm{where\: l \, =\, \sqrt{(r^{2} + h^{2}))}}$$
Example
Height = 7, Radius = 5
Volume of cone = 183.33333333333331
Area of cone = 213.75082562495555
Explanation
Volume of cone = (⅓) * π * (r)2* h
= (⅓) * (22/7) * (5*5) * 7
= 183.33333333333331
For the area of the cone, first calculate the value of ‘l’
Length ‘l’ = √(r2 + h2))
= √(52 + 72))
= 8.602
Now putting the value of ‘l’ in the formula for area of the cone-
Area of cone =π* r * (r + l)
= (22/7) * 5 * (5 + 8.602)
= 213.75082562495555
Calculating the volume and area of a cone
Algorithm
Step 1 − Declare a variable for storing the height of the cone- ‘h’.
Step 2 − Declare a variable for storing the radius of the cone- ‘r’.
Step 3 − Declare two variables for storing the area of the cone- ‘area’ and volume of the cone- ‘volume’ and initialize both the variables with the value 0.
Step 4 − Calculate the volume using the formula- Volume = π * (r)2 * h, and store it in the ‘volume’ variable in the function calculateVolumeOfCone().
Step 5 − Calculate the value of ‘l’ using √(r2 + h2)) so that it can be used in the formula for the area of the cone.
Step 6 − Calculate the area using the formula- Area = π * r * (r + l), and store it in the ‘area’ variable in the function calculateAreaOfCone().
Step 7 − Print the calculated volume and area of the cone, i.e, the value stored in the ‘volume’ and ‘area’ variables
Example
package main // fmt package allows us to print formatted strings import ( "fmt" "math" ) func calculateVolumeOfCone(h, r float64) float64 { // declaring variable ‘volume’ for storing the volume of the cone var volume float64 = 0 // calculating the volume of the cone using its formula volume = (1.0 / 3.0) * (22.0 / 7.0) * r * r * h return volume } func calculateAreaOfCone(h, r float64) float64 { // declaring variable ‘area’ for storing the area of the cone var area float64 = 0 // calculating the value of length 'l' l := math.Sqrt((r * r) + (h * h)) // calculating the area of the cone using its formula area = (22.0 / 7.0) * r * (r + l) return area } func main() { // declaring variable ‘height’ for storing the height of the cone var h float64 = 7 // declaring variable ‘radius’ for storing the radius of the cone var r float64 = 5 // declaring variables ‘area’ and ‘volume’ for storing the area and volume of the cone var area, volume float64 fmt.Println("Program to calculate the volume and area of the Cone \n") // calling function calculateVolumeOfCone() for // calculating the volume of the cone volume = calculateVolumeOfCone(h, r) // calling function calculateAreaOfCone() for calculating // the area of the cone area = calculateAreaOfCone(h, r) // printing the results fmt.Println("Height of the cone : ", h) fmt.Println("Radius of the cone : ", r) fmt.Println("Therefore, Volume of cone : ", volume) fmt.Println("Area of cone : ", area) }
Output
Program to calculate the volume and area of the Cone Height of the cone : 7 Radius of the cone : 5 Therefore, Volume of cone : 183.33333333333331 Area of cone : 213.75082562495555
Description of code
var h float64 = 7, var r float64 = 5 − In this line, we are declaring the variables for height ‘h’ and radius ‘r’.
calculateVolumeOfCone(h, r float64) float64 − This is the function where we are calculating the volume of the cone. The function has the variable ‘h’ and ‘r’ of data type float64 as the parameter and also has a return type of float64.
volume = (1.0 / 3.0) * (22.0 / 7.0) * r * r * h − If we desire the output to be of data type float, we explicitly need to convert the values, that is the reason we are using values 22.0 and 7.0 instead of 22 and 7. Using the above formula, we can calculate the volume of the cone.
return volume − for returning the volume of the cone.
volume = calculateVolumeOfCone(h, r) − We are calling the function calculateVolumeOfCone() and storing the calculated value in the ‘volume’ variable in the main method.
calculateAreaOfCone(h, r float64) float64 − This is the function where we are calculating the area of the cone. The function has the variable ‘h’ and ‘r’ of data type float64 as the parameter and also has a return type of float64.
l := math.Sqrt((r * r) + (h * h)) − We will need the value of length ‘l’ for calculating the area of the cone.
area = (22.0 / 7.0) * r * (r + l) − Using this formula, we calculate the area of the cone.
return area − for returning the area of the cone
area = calculateAreaOfCone(h, r) − We are calling the function calculateAreaOfCone() and storing the calculated value in the ‘area’ variable.
Conclusion
This is all about calculating the volume and area of a cone using Go programming. We have also maintained code modularity by using separate functions for calculating the area and volume which also increases the code reusability. You can explore more about Golang programming using these tutorials.