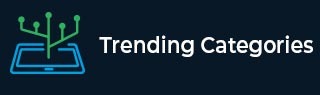
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Golang program to calculate the sum of right diagonal elements
In this article, we will learn how to calculate the sum of right diagonal matrix with the help of different examples. A matrix is a 2-d array. The output will be printed on the screen using fmt.println() function which is a print statement in Golang.
Algorithm
Step 1 − Create a package main and declare fmt(format package) package in the program where main produces executable codes and fmt helps in formatting input and output.
Step 2 − create a function main, in that function create a matrix, and fill with some values inside it.
Step 3 − Print the matrix on the console using the print statement in Golang.
Step 4 − Create a variable sum and initialize it with the value zero and this variable is used to store the sum of the matrix.
Step 5 − Run a loop till the length of the matrix such that i=0 and i<len(matrix_val) and keep adding the right diagonal elements in the sum on every iteration.
Step 6 − on the termination of the loop, the sum received is printed on the screen.
Step 7 − The print statement is executed using fmt.Println() function where ln means new line.
When Size of the Matrix is Known
In this example, we will use a for loop to calculate sum of right diagonal matrix, here the size of matrix is known. A sum variable will be used to store the value and the output will be printed on the console using print statement in Golang.
Example
package main import "fmt" func main() { matrix_val := [][]int{{10, 20, 30}, {40, 50, 60}, {70, 80, 90}} //create matrix fmt.Println("The matrix given here is:", matrix_val) // Initialize sum to 0 sum := 0 for i := 0; i < len(matrix_val); i++ { sum += matrix_val[i][len(matrix_val)-i-1] } fmt.Println("Sum of right diagonal elements:", sum) }
Output
The matrix given here is: [[10 20 30] [40 50 60] [70 80 90]] Sum of right diagonal elements: 150
When Size of the Matrix is Not Known
In this example, we will use a for loop to calculate the sum of right diagonal matrix, here the size of the matrix is not known. A sum variable will be used to store the value and the output will be printed on the console using the print statement in Golang.
Example
package main import "fmt" func main() { matrix_val := [][]int{{10, 20, 30}, {40, 50, 60}, {70, 80, 90}} fmt.Println("The matrix originally created is:", matrix_val) sum := 0 // length of the matrix length := len(matrix_val) // Iterate through columns for i := 0; i < length; i++ { sum += matrix_val[i][length-i-1] } fmt.Println("The Sum of right diagonal matrix is:", sum) }
Output
The matrix originally created is: [[10 20 30] [40 50 60] [70 80 90]] The Sum of right diagonal matrix is: 150
Using Nested For Loop
In this example we will use a nested for loop to calculate sum of right diagonal matrix. A sum variable will be used to store the value and the output will be printed on the console using print statement in Golang.
Example
package main import "fmt" func main() { var matrix_val [3][3]int = [3][3]int{{10, 20, 30}, {40, 50, 60}, {70, 80, 90}} var sum int = 0 fmt.Println("The original matrix is:", matrix_val) for i, row := range matrix_val { //run nested for loop for j, value := range row { if i == j { sum += value } } } fmt.Println("Sum of right diagonal elements of matrix is:", sum) //print sum }
Output
The original matrix is: [[10 20 30] [40 50 60] [70 80 90]] Sum of right diagonal elements of matrix is: 150
Conclusion
In the above program, we used three examples to calculate the sum of right diagonal elements of a slice. In the first example we used for loop to calculate sum when size of matrix is known whereas in the second example, we used the same logic to calculate sum but that case will be used when the size of matrix is not known. In the third example we used nested for loop. Hence, program executed successfully.