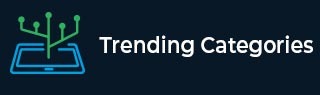
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Golang program to calculate the sum of all odd numbers up to N
In this tutorial, we will be discussing the program to find the sum of all odd numbers up to ‘N’ in Golang programming.
But before writing the code for this, let’s have a brief discussion on odd numbers.
Odd Numbers
An odd number is a number that is not completely divisible by 2 and its remainder is always 1 when the number is divided by 2. A simple way to check whether a number is an odd number or not is to check its last digit. If the last digit is 1, 3, 5, 7, or 9, then the number is said to be an odd number.
Approach 1: Iterate through the first n odd numbers and add them
Example
- Number = 4
Sum of first 4 odd numbers = 16
First 4 odd numbers are- 1, 3, 5, and 7
Sum of these numbers = 1 + 3 + 5 + 7
= 16
Algorithm
Step 1 − Declare a variable ‘N’ for storing the number.
Step 2 − Declare a variable ‘nextOdd’ for storing the number 1.
Step 3 − Declare a variable ‘sum’ for storing the sum of odd numbers and initialize it with 0.
Step 4 − Create a for loop starting from 1 and run it till it is less than or equal to ‘N’.
Step 5 − Calculate the sum by adding the next odd number back to the sum until the for loop ends.
Step 6 − All odd numbers have a difference of 2. Therefore, calculate the next odd number by adding 2 to the existing number.
Step 7 − Once the for loop ends, print the result stored in ‘sum’.
Example
package main // fmt package allows us to print formatted strings import "fmt" func main() { fmt.Println("Program to calculate the sum of all odd numbers up to N \n") // declaring variable ‘N’ for storing the number var N int = 10 // declaring variable ‘nextOdd’ for storing the next odd number var nextOdd int = 1 // declaring variable ‘sum’ for storing the sum of all odd numbers up to N var sum int = 0 for x := 1; x <= N; x++ { // calculating the I’m sum of odd numbers sum = sum + nextOdd // calculating the next odd number nextOdd = nextOdd + 2 } fmt.Println("Finding the sum of first", N, "Odd numbers") fmt.Println("Therefore, Sum of all odd numbers up to N : ", sum) }
Output
Program to calculate the sum of all odd numbers up to N Finding the sum of first 10 Odd numbers Therefore, Sum of all odd numbers up to N : 100
Description of code
var nextOdd int = 1 − We will be storing the value of the next odd number in this variable. Initially, we assigned the value 1 to it because the odd numbers start from the number 1.
var sum int = 0 − We will be storing the resultant sum of the odd numbers in his variable and will initialize it with 0 in the beginning.
for x := 1; x <= N; x++ − This for loop will be used to count the sum of the odd numbers. It will start from 1 and will run until the value of ‘x’ is less than or equal to ‘N’.
sum = sum + nextOdd − In this, we are calculating the sum by adding the next odd number back to sum until the for loop ends.
nextOdd = nextOdd + 2 − This is used to calculate the next odd number. All odd numbers have a difference of 2, that is why we are adding 2 in order to generate the next odd number.
fmt.Println("Therefore, Sum of odd numbers up to N : ", sum) − printing the calculated sum of all odd numbers until ‘N’.
Approach 2: Using the formula for calculating the sum of all odd numbers
Formula
$$\mathrm{Sum\: of\: first\: 'n' \: odd \: numbers\, =\, n \ast n}$$
Example
Number = 10
Sum of first 10 odd numbers = N * N
= 10 * 10
= 100
Algorithm
Step 1 − Declare a variable for storing the number- ‘N’.
Step 2 − Declare a variable for storing the sum of all odd numbers- ‘sum’ and initialize it with 0.
Step 3 − Calculate the sum using the formula N * N and store it in the ‘sum’ variable in the function calculateSumOfOddNumbers().
Step 4 − Print the calculated sum, i.e, the value stored in the variable ‘sum’.
Example
package main // fmt package allows us to print formatted strings import "fmt" func calculateSumOfOddNumbers(N int) int { // declaring variable ‘sum’ for storing the sum of all odd numbers var sum int = 0 // calculating the sum sum = N * N return sum } func main() { // declaring variable ‘N’ for storing the number var N int = 10 var sum int fmt.Println("Program to calculate the sum of all odd numbers up to N \n") // calling function calculateSumOfOddNumbers() for // calculating the sum of all odd numbers sum = calculateSumOfOddNumbers(N) // printing the results fmt.Println("Finding the sum of first", N,"Odd numbers") fmt.Println("Therefore, Sum of all odd numbers up to N : ", sum) }
Output
Program to calculate the sum of all odd numbers up to N Finding the sum of first 10 Odd numbers Therefore, Sum of all odd numbers up to N : 100
Description of code
calculateSumOfOddNumbers(N int) int − This is the function where we are calculating the sum of odd numbers. The function has the variable ‘N’ of data type int as the parameter and also has a return type value of data type int.
sum = N * N − We are finding the sum of odd numbers using this formula- N * N.
return sum − for returning the calculated sum of all odd numbers.
sum = calculateSumOfOddNumbers(N) − We are calling the function calculateSumOfOddNumbers() and storing the calculated value in the ‘sum’ variable.
fmt.Println("Therefore, Sum of odd numbers : ", sum) − printing the calculated sum of all odd numbers.
Conclusion
This is all about calculating the sum of odd numbers up to N using two approaches. The second approach is better in terms of time complexity and modularity. You can explore more about Golang programming using these tutorials.