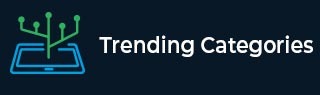
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Golang Program to Calculate The Power using Recursion
In this tutorial, we will learn how to calculate the power using recursion technique in Go programming language.
Power can be defined as a number being multiplied by itself a specific number of times.
Exponent can be defined to the number of times a number is used in a multiplication. Powers and exponents are important tools to rewrite long multiplication problems in mathematics, especially in algebra.
Example: 24 = 2 × 2 × 2 × 2 = 16, where 2 is the base and 4 is the exponent.
A Recursion is where a function calls itself by direct or indirect means. Every recursive function has a base case or base condition which is the final executable statement in recursion and halts further calls.
Below we have shown examples with two different kinds of recursion methods.
Example 1: Golang Program Code to calculate the power using Direct Recursion Method
Syntax
Result = (num * POWER(num, power-1) // Recursive function call to the function POWER() by itself up to the defined condition
Algorithm
Step 1 − Import the package fmt.
Step 2 − Create the function POWER().
Step 3 − We will use an if-conditional statement.
Step 4 − Recursive call to the function itself.
Step 5 − Start the function main().
Step 6 − Declare and initialize the variables.
Step 7 − Call the function POWER ().
Step 8 − Print the result on the screen using fmt.Printf().
Example
// GOLANG PROGRAM TO CALCULATE THE POWER USING RECURSION // Direct Recursion example package main // fmt package provides the function to print anything import "fmt" // create a function func POWER(num int, power int) int { var result int = 1 if power != 0 { // Recursive function call to itself result = (num * POWER(num, power-1)) } return result } func main() { fmt.Println("Golang Program to calculate the power using recursion") // declare and initialize the integer variables var base int = 4 var power int = 2 var result int // calling the POWER() function result = POWER(base, power) // Print the result using in-built function fmt.Printf() fmt.Printf("%d to the power of %d is: %d\n", base, power, result) }
Output
Golang Program to calculate the power using recursion 4 to the power of 2 is: 16
Description of the Code
In the above program, we first declare the package main.
We imported the fmt package that includes the files of package fmt
Next we create a function POWER() to calculate the power using direct recursion technique
We will use an if conditional statement which allows you to execute one block of code if the specified condition is true and then recursive call to the function itself
Now start the function main().GO program execution starts with the function main(). Declare the integer variables base, power and result
Now calling the POWER() function
And finally printing the result on the screen using in-built function fmt.Printf().This function is defined under the fmt package and it helps to write standard output.
Example 2: Golang Program code to calculate the Power using Indirect Recursion Method
Syntax
func recursion_1() { recursion_2()} func recursion_2(){ recursion_1()} func main() { recursion_1(); }
Algorithm
Step 1 − Import the package fmt.
Step 2 − Create the function POWER_1().
Step 3 − We will use an if−conditional statement.
Step 4 − Recursive call to the function POWER_2().
Step 5 − Create the function POWER_2().
Step 6 − Recursive call to the function POWER_1() indirectly.
Step 7 − Start the function main().
Step 8 − Declare and initialize the variables.
Step 9 − Call the function POWER_2 ().
Step 10 − Print the result on the screen using fmt.Printf().
Example
// GOLANG PROGRAM TO CALCULATE THE POWER USING RECURSION // Indirect Recursion example package main // fmt package provides the function to print anything import "fmt" // create a first Recursive function func POWER_1(num int, power int) int { var result int = 1 if power != 0 { // Recursive function call to the second function result = (num * POWER_2(num, power-1)) } return result } // create a second Recursive function func POWER_2(num int, power int) int { var result int = 1 if power != 0 { // Recursive function call to the first function // which calls this first function indirectly result = (num * POWER_1(num, power-1)) } return result } func main() { fmt.Println("Golang Program to calculate the power using recursion") // declare and initialize the integer variables var base int = 5 var power int = 2 var result int // calling the POWER_2() function result = POWER_2(base, power) // Print the result using in-built function fmt.Printf() fmt.Printf("%d to the power of %d is: %d\n", base, power, result) }
Output
Golang Program to calculate the power using recursion 5 to the power of 2 is: 25
Description of the Code
In the above program, we first declare the package main.
We imported the fmt package that includes the files of package fmt.
Next we create a function POWER_1() to calculate the power using indirect recursion technique.
We will use an if conditional statement which allows you to execute one block of code if the specified condition is true and then recursive call to the second function POWER_2().
Next we create a function POWER_2 (). Here recursive function call to the first function is made which calls the first function POWER_1() indirectly.
Now start the function main().GO program execution starts with the function main().
Declare the integer variables base, power and result.
Now calling the POWER_2() function.
And finally printing the result on the screen using in-built function fmt.Printf().This function is defined under the fmt package and it helps to write standard output.
Conclusion
In the above two examples we have successfully complied and executed Golang Program code to calculate the power using the recursion technique. In the first example we have shown direct recursion method and in the second example we have shown indirect recursion method.