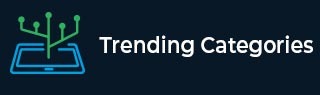
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Golang Program to Calculate Difference Between Two Time Periods
In this tutorial, we will write a golang programs to calculate the difference between two time periods given in the following programs. To get the time difference between two time periods we can either use a library function or can create a separate user-defined function to achieve the results.
Method 1: Calculate the Difference between Two Time Periods using Internal Function
In this method, we will write a go language program to find the difference between time periods using pre-defined functions in go programming language.
Syntax
func (t Time) Sub(u Time) Duration
The sub() function in go is used to get the difference between two dates. In this function the first two parameters i.e., t and u are date values and this function returns the difference between two values in hours, minutes, and seconds.
Algorithm
Step 1 − First, we need to import the fmt and time. The time package allows us to use other predefined packages like time.Date().
Step 2 − Start the main() function.
Step 3 − Initialize firstDate and secondDate variables by passing the dates and times in time.Date() function in the order of yy/mm/dd/hrs//min//sec
Step 4 − Find the difference between the given dates using Sub() function this function takes the second date as an argument and calculates the required difference.
Step 5 − Print the result on the screen in various formats.
Step 6 − We can print the years, days, months, weeks, hours, second, milliseconds, etc.
Example
Golang program to calculate the difference between two time periods using internal function
package main import ( "fmt" "time" ) func main() { firstDate := time.Date(2022, 4, 13, 3, 0, 0, 0, time.UTC) secondDate := time.Date(2010, 2, 12, 6, 0, 0, 0, time.UTC) difference := firstDate.Sub(secondDate) fmt.Println("The difference between dates", firstDate, "and", secondDate, "is: ") fmt.Printf("Years: %d\n", int64(difference.Hours()/24/365)) fmt.Printf("Months: %d\n", int64(difference.Hours()/24/30)) fmt.Printf("Weeks: %d\n", int64(difference.Hours()/24/7)) fmt.Printf("Days: %d\n", int64(difference.Hours()/24)) fmt.Printf("Hours: %.f\n", difference.Hours()) fmt.Printf("Minutes: %.f\n", difference.Minutes()) fmt.Printf("Seconds: %.f\n", difference.Seconds()) fmt.Printf("Nanoseconds: %d\n", difference.Nanoseconds()) }
Output
The difference between dates 2022-04-13 03:00:00 +0000 UTC and 2010-02-12 06:00:00 +0000 UTC is: Years: 12 Months: 148 Weeks: 634 Days: 4442 Hours: 106629 Minutes: 6397740 Seconds: 383864400 Nanoseconds: 383864400000000000
Method 2: Calculate the Difference between Two Time Periods using a User-Defined Function
In this method, we will create a different function to calculate the difference between the two provided dates. The function will take the two dates as arguments and return the respective result.
Algorithm
Step 1 − First, we need to import the fmt and time packages. The time package allows us to use other predefined functions like time.Date().
Step 2 − Create the leapYear() function to calculate the number of leap years between the two dates.
Step 3 − Also create the getDifference() to get the difference between date and time. getDifference() function returns the days, hours, minutes, and seconds.
Step 4 − Start the main() function.
Step 5 − Initialize date1 and date2 variables by passing the dates and times in time.Date() function in the order of yy/mm/dd/hrs//min//sec.
Step 6 − Swap the two dates if date1 occurs after date2.
Step 7 − Call the getDifference() by passing the two dates as arguments to the function.
Step 8 − Store the result obtained by the function in a different variable and print them on the screen in different formats using fmt.Println() function.
Example
Golang program to calculate the difference between two time periods using a user-defined function
package main import ( "fmt" "time" ) func leapYears(date time.Time) (leaps int) { y, m, _ := date.Date() if m <= 2 { y-- } leaps = y/4 + y/400 - y/100 return leaps } func getDifference(a, b time.Time) (days, hours, minutes, seconds int) { monthDays := [12]int{31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31} y1, m1, d1 := a.Date() y2, m2, d2 := b.Date() h1, min1, s1 := a.Clock() h2, min2, s2 := b.Clock() totalDays1 := y1*365 + d1 for i := 0; i < (int)(m1)-1; i++ { totalDays1 += monthDays[i] } totalDays1 += leapYears(a) totalDays2 := y2*365 + d2 for i := 0; i < (int)(m2)-1; i++ { totalDays2 += monthDays[i] } totalDays2 += leapYears(b) days = totalDays2 - totalDays1 hours = h2 - h1 minutes = min2 - min1 seconds = s2 - s1 if seconds < 0 { seconds += 60 minutes-- } if minutes < 0 { minutes += 60 hours-- } if hours < 0 { hours += 24 days-- } return days, hours, minutes, seconds } func main() { date1 := time.Date(2020, 4, 27, 23, 35, 0, 0, time.UTC) date2 := time.Date(2018, 5, 12, 12, 43, 23, 0, time.UTC) if date1.After(date2) { date1, date2 = date2, date1 } days, hours, minutes, seconds := getDifference(date1, date2) fmt.Println("The difference between dates", date1, "and", date2, "is: ") fmt.Printf("%v days\n%v hours\n%v minutes\n%v seconds", days, hours, minutes, seconds) }
Output
The difference between dates 2018-05-12 12:43:23 +0000 UTC and 2020-04-27 23:35:00 +0000 UTC is: 716 days 10 hours 51 minutes 37 seconds
Conclusion
We have successfully compiled and executed a go language program to get the difference between two time periods along with examples. In the first example, we used internal functions and in the second example, we used a user-defined function to obtain the results.