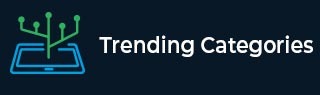
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Golang program to add elements at first and last position of linked list
In golang, a linked list is a unique data structure in which there is a value in the node and the next pointer which points to the next node. The list's initial node is referred to as the head, while the list's last node which points to nil depicts the end of list. We will add elements at the first and last position of the linked list using two examples. In the first example node struct will be used and in the second example ListNode struct will be used.
Method 1: Using Node Struct
In this method, we will use node struct to add elements at given position in linked list. Here, we will create two methods addfirst and addlast method where the program will be written to add the elements.
Algorithm
Step 1 − Create a package main and declare fmt(format package) package in the program where main produces executable codes and fmt helps in formatting input and output.
Step 2 − Make a Node structure with two fields − data, which will hold the node's value, and next, which will hold a pointer to the node after it in the list.
Step 3 − Make an addFirst function. A node should be added to the linked list's front first. The list's head and the data to be added are the two inputs for this function. With the supplied data, the function generates a new node and sets its next field to the top of the list as it is at the moment.
Step 4 − The function returns a pointer to the new node, which takes over as the list's new head.
Step 5 − To add a node to the end of the linked list, create the function addLast.
Step 6 − The list's head and the data to be added are the two inputs for this function. The function iterates through the list beginning at the top and continues until it reaches the bottom.
Step 7 − Then, the next field of the current node is then set to point to the new node after creating a new node with the supplied data. The function returns the list's head, which is left alone.
Step 8 − To print the linked list's elements, create the function printList. This method iterates through the list while printing the value of each node's data field. It takes the list's head as a parameter.
Step 9 − Then create the list's head node in the main function, and call the addFirst and addLast functions to add elements to the list's first and last nodes, respectively.
Step 10 − Finally, use the printList method to show the connected list's elements.
Example
In this example, we used node struct to add elements at first and last position of linked list.
package main import "fmt" type Node struct { //create a node struct data_val int next *Node } func addFirst(head *Node, data_val int) *Node { //create addFirst to add element at first place newNode := &Node{data_val: data_val} newNode.next = head return newNode } func addLast(head *Node, data_val int) *Node { //create addLast to add element at last place newNode := &Node{data_val: data_val} current := head for current.next != nil { current = current.next } current.next = newNode return head } func printList(head *Node) { current := head for current != nil { fmt.Printf("%d -> ", current.data_val) current = current.next } fmt.Println("nil") } func main() { head := &Node{data_val: 20} fmt.Println("The first and last elements are added as follows:") head = addFirst(head, 10) head = addLast(head, 30) printList(head) //print the list }
Output
The first and last elements are added as follows: 10 -> 20 -> 30 -> nil
Method 2: Using ListNode Struct
In this method, we will use ListNode struct to add elements at given position in linked list. Here, we will create two methods addfirst and addlast method where the program will be written to add the elements.
Algorithm
Step 1 − Create a package main and declare fmt(format package) package in the program where main produces executable codes and fmt helps in formatting input and output.
Step 2 − Build a structure and the ListNode contains two fields − next to store a pointer to the next node in the list and data_val to hold the node's value.
Step 3 − Create an addFirst function. A node should be added to the linked list's front first. The list's head and the value to be added are the two inputs for this function.
Step 4 − In the next step the function takes a value and produces a new node, setting the next field to the head of the list as it is at that moment. The function returns a pointer to the new node, which takes over as the list's new head.
Step 5 − To add a node to the end of the linked list, create the function addLast. The list's head and the value to be added are the two inputs for this function.
Step 6 − The function iterates through the list beginning at the top and continues until it reaches the bottom.
Step 7 − The next field of the current node is then set to point to the new node after creating a new node with the specified value. The function returns the list's head, which is left alone.
Step 8 − To print the linked list's elements, create the function printList. This function prints the value of each node's val field after iterating through the list using the list's head as an argument.
Step 9 − Create the list's head node in the main function, then call the addFirst and addLast functions to add elements to the list's first and last nodes, respectively.
Step 10 − Finally, use the printList method to show the connected list's elements.
Example
In this example, we will use ListNode struct to add elements at first and last position of linked list.
package main import "fmt" type ListNode struct { //create listnode struct data_val int next *ListNode } func addFirst(head *ListNode, data_val int) *ListNode { //create addFirst function to add element at first place newNode := &ListNode{data_val: data_val} newNode.next = head return newNode } func addLast(head *ListNode, data_val int) *ListNode { //create addLast function to add element at last place newNode := &ListNode{data_val: data_val} current := head for current.next != nil { current = current.next } current.next = newNode return head } func printList(head *ListNode) { current := head for current != nil { fmt.Printf("%d -> ", current.data_val) current = current.next } fmt.Println("nil") } func main() { head := &ListNode{data_val: 1} head = addFirst(head, 0) head = addLast(head, 2) fmt.Println("The first and last elements are added as follows:") printList(head) //print the linked list }
Output
The first and last elements are added as follows: 0 -> 1 -> 2 -> nil
Conclusion
We execute the program of adding the elements at the first and last position of the linked list using two examples. In the first example, we used node struct and in the second example, we used Listnode struct.