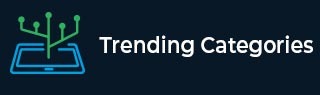
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Golang program to access elements from a linked list
In Go Programming language, a linked list is a data structure which contains a node that further contains two values, the data and the next, where next points to the next node in the list. We will use two methods in this program to access elements from a linked list. In the first example iteration will be used and in the second example a variable current will be used to access the elements.
Method 1: Using Iteration
This program builds a linked list with three members and iterates through it to access and output each element's value. The output will be a linked list with pre-defined elements. Let’s see the code and the algorithm to understand the concept.
Algorithm
Step 1 − Create a package main and declare fmt(format package) package in the program where main produces executable codes and fmt helps in formatting input and output.
Step 2 − Create a Node struct having two fields, value_num and next, each of which contains a pointer to the following node in the linked list. Value contains the data.
Step 3 − Create a head node with the value 1 in the main function.
Step 4 − Set a new node with the value 2 as the next field of the head node.
Step 5 − Set a new node with the value 3 as the second node's next field.
Step 6 − Set the head node with the value of the variable node you just created.
Step 7 − Iterate through the linked list using a for loop. The loop will continue until the node variable equals nil, which denotes the end of the linked list, according to the loop condition node!= nil.
Step 8 − Print the value of current node in the loop’s body using fmt.Println(node.value_num).
Step 9 − Use node = node.next to update the node variable to the subsequent node in the linked list.
Step 10 − Repeat steps 6 through 8 until the end of linked list.
Example
In this example we will use iteration to access the elements of a linked list. Let’s see through the code.
package main import "fmt" // Node represents a node in a linked list type Node struct { value_num int next *Node } //create main function to execute the program func main() { head := &Node{value_num: 10} head.next = &Node{value_num: 20} head.next.next = &Node{value_num: 30} // Accessing elements from linked list fmt.Println("Accessing elements of linked list:") node := head for node != nil { fmt.Println(node.value_num) node = node.next } }
Output
Accessing elements of linked list: 10 20 30
Method 2: Using Current Variable
This method behaves similar like the method discussed before, here we only use a variable current instead of a node to traverse the linked list. In a linked list, the variable current starts at the head and is updated after each iteration to refer to the subsequent node. When current reaches zero, the linked list is said to be reached its conclusion.
Algorithm
Step 1 − Create a package main and declare fmt(format package) package in the program where main produces executable codes and fmt helps in formatting input and output.
Step 2 − Create a Node struct having two fields, value_num and next, each of which contains a pointer to the following node in the linked list. Value contains the data.
Step 3 − Create a head node with the value 10 in the main function.
Step 4 − Set a new node with the value 20 as the next field of the head node.
Step 5 − Set a new node with the value 30 as the second node's next field.
Step 6 − Set the head node as the equal of a variable current.
Step 7 − Iterate through the linked list using a for loop. Since the linked list's end is indicated by the conditional statement current!= nil, the loop will keep running until the current variable equals nil.
Step 8 − Print the value of current node in loop’s body using fmt.Println(current.value).
Step 9 − Using current = current, move the current variable to the following node in the linked list.
Step 10 − Repeat steps 6 through 8 till the end of linked list.
Example
In this example, we will use a current variable in iteration.
package main import "fmt" // Node represents a node in a linked list type Node struct { value_num int next *Node } //create main function to execute the program func main() { head := &Node{value_num: 10} head.next = &Node{value_num: 20} head.next.next = &Node{value_num: 30} // Accessing elements from linked list fmt.Println("Accessing elements of linked list:") current := head for current != nil { fmt.Println(current.value_num) current = current.next } }
Output
Accessing elements of linked list: 10 20 30
Conclusion
We executed the program of accessing the elements of linked list using two examples. In the first example we iterated through the linked list and in the second example we used a current variable to iterate through the LinkedList. Both the programs give similar output. Hence, the program executed successfully.