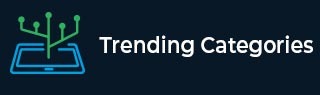
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Golang Pointer to an Array as Function Argument
Golang allows us to use pointers to access the value of an object in memory. Using pointers can improve the performance of the code by reducing the memory allocation and enhancing the efficiency of the program. In this article, we will explore how to use a pointer to an array as a function argument in Golang.
Pointer to an Array in Golang
In Golang, an array is a fixed-length data structure that stores a collection of elements of the same type. When we pass an array to a function as an argument, a copy of the array is created, and any changes made to the array within the function are not reflected outside the function. To modify the original array, we can pass a pointer to the array as an argument to the function.
To declare a pointer to an array, we use the following syntax −
var ptr *[size]type
Here, ptr is the pointer to the array, and size is the size of the array. The type represents the data type of the array.
Passing a Pointer to an Array as Function Argument
To pass a pointer to an array as a function argument, we need to declare the function with a pointer argument. The syntax for declaring a pointer argument is as follows −
func functionName(ptr *[size]type)
Here, functionName is the name of the function, ptr is the pointer to the array, size is the size of the array, and type is the data type of the array.
Let's look at an example of passing a pointer to an array as a function argument in Golang.
Example
In this example, we will create an array of integers, and then we will pass a pointer to this array to a function. The function will modify the array by doubling each element.
package main import "fmt" func doubleArray(arr *[5]int) { for i := 0; i < len(arr); i++ { arr[i] *= 2 } } func main() { arr := [5]int{1, 2, 3, 4, 5} fmt.Println("Array before function call:", arr) doubleArray(&arr) fmt.Println("Array after function call:", arr) }
Output
Array before function call: [1 2 3 4 5] Array after function call: [2 4 6 8 10]
Explanation
In the above code, we have defined an array of integers with 5 elements, i.e., [1, 2, 3, 4, 5]. We passed a pointer to this array to the doubleArray function by calling it with &arr. Inside the doubleArray function, we have doubled each element of the array. Finally, we have printed the modified array after the function call.
Conclusion
Using pointers to an array can improve the performance of the code by reducing the memory allocation and enhancing the efficiency of the program. We can pass a pointer to an array as a function argument to modify the original array. In this article, we learned how to use a pointer to an array as a function argument in Golang.