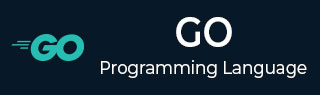
- Go Tutorial
- Go - Home
- Go - Overview
- Go - Environment Setup
- Go - Program Structure
- Go - Basic Syntax
- Go - Data Types
- Go - Variables
- Go - Constants
- Go - Operators
- Go - Decision Making
- Go - Loops
- Go - Functions
- Go - Scope Rules
- Go - Strings
- Go - Arrays
- Go - Pointers
- Go - Structures
- Go - Slice
- Go - Range
- Go - Maps
- Go - Recursion
- Go - Type Casting
- Go - Interfaces
- Go - Error Handling
- Go Useful Resources
- Go - Questions and Answers
- Go - Quick Guide
- Go - Useful Resources
- Go - Discussion
Go - Passing pointers to functions
Go programming language allows you to pass a pointer to a function. To do so, simply declare the function parameter as a pointer type.
In the following example, we pass two pointers to a function and change the value inside the function which reflects back in the calling function −
package main import "fmt" func main() { /* local variable definition */ var a int = 100 var b int = 200 fmt.Printf("Before swap, value of a : %d\n", a ) fmt.Printf("Before swap, value of b : %d\n", b ) /* calling a function to swap the values. * &a indicates pointer to a ie. address of variable a and * &b indicates pointer to b ie. address of variable b. */ swap(&a, &b); fmt.Printf("After swap, value of a : %d\n", a ) fmt.Printf("After swap, value of b : %d\n", b ) } func swap(x *int, y *int) { var temp int temp = *x /* save the value at address x */ *x = *y /* put y into x */ *y = temp /* put temp into y */ }
When the above code is compiled and executed, it produces the following result −
Before swap, value of a :100 Before swap, value of b :200 After swap, value of a :200 After swap, value of b :100
go_pointers.htm
Advertisements