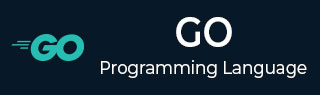
- Go Tutorial
- Go - Home
- Go - Overview
- Go - Environment Setup
- Go - Program Structure
- Go - Basic Syntax
- Go - Data Types
- Go - Variables
- Go - Constants
- Go - Operators
- Go - Decision Making
- Go - Loops
- Go - Functions
- Go - Scope Rules
- Go - Strings
- Go - Arrays
- Go - Pointers
- Go - Structures
- Go - Slice
- Go - Range
- Go - Maps
- Go - Recursion
- Go - Type Casting
- Go - Interfaces
- Go - Error Handling
- Go Useful Resources
- Go - Questions and Answers
- Go - Quick Guide
- Go - Useful Resources
- Go - Discussion
Go - The goto Statement
A goto statement in Go programming language provides an unconditional jump from the goto to a labeled statement in the same function.
Note − Use of goto statement is highly discouraged in any programming language because it becomes difficult to trace the control flow of a program, making the program difficult to understand and hard to modify. Any program that uses a goto can be rewritten using some other construct.
Syntax
The syntax for a goto statement in Go is as follows −
goto label; .. . label: statement;
Here, label can be any plain text except Go keyword and it can be set anywhere in the Go program above or below to goto statement.
Flow Diagram
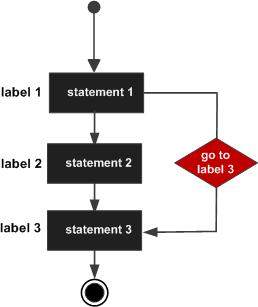
Example
package main import "fmt" func main() { /* local variable definition */ var a int = 10 /* do loop execution */ LOOP: for a < 20 { if a == 15 { /* skip the iteration */ a = a + 1 goto LOOP } fmt.Printf("value of a: %d\n", a) a++ } }
When the above code is compiled and executed, it produces the following result −
value of a: 10 value of a: 11 value of a: 12 value of a: 13 value of a: 14 value of a: 16 value of a: 17 value of a: 18 value of a: 19
go_loops.htm
Advertisements