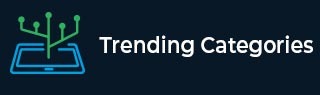
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Given a sorted array of 0’s and 1’s, find the transition point of the array in C++
Given an array of sorted numbers containing only 0s and 1s, find the transition point. A transition point is the index of the first ‘1’ appearing in the array. For example,
Input-1 −
N = 6 arr[ ] = {0,0,0,0,1,1}
Output −
4
Explanation − Since in the given array containing 0’s and 1’s we can see that the element at the index ‘4’ is having the number ‘1’.
Input-2 −
N = 5 arr[ ] = {0,0,1,1,1}
Output −
2
Explanation − In the given array containing 0’s and 1’s, we can see that the element at the index ‘2’ is having the number ‘1’. Thus, we will return 2.
Approach to Solve this Problem
In the given array of integers, we have to find the index of the first 1. To solve this particular problem, we can solve it using the Binary Search Method to find the index of the first ‘1’.
Take Input an array of N Binary Numbers
Now a function transitionPoint(int *arr, int n) takes an array as input and its size and returns the index of the first ‘1’ appearing in the array.
Take two pointers low, high which are initialized as ‘0’ and ‘1’.
Now we will find the middle point of the array and will check if it is ‘1’ or not.
If the middle of the array is ‘1’ then we will return its index otherwise we will check by moving ahead.
Increment the low pointer and again check for the ‘1’.
Repeat the steps until we don’t get ‘1’.
Example
#include <bits/stdc++.h> using namespace std; int transitionPoint(int *arr, int n){ int low=0; int high= n-1; while(low<=high){ int mid = (low+high)/2; if(arr[mid]==0) low= mid+1; else if(arr[mid]==1){ if(mid==0 || (mid>0 && arr[mid-1]==0)) return mid; high= mid-1; } } return -1; } int main(){ int n= 6; int arr[n]= {0,0,0,1,1,1}; int ans= transitionPoint(arr,n); if(ans>=0){ cout<<"Transition Point is:"<<ans<<endl; } else{ cout<<"Not Found"<<endl; } return 0; }
Output
Running the above code will generate the output as,
Transition Point is: 3
The given array {0,0,0,1,1,1} has element ‘1’ present at index ‘3’, thus we get the output as ‘3’.