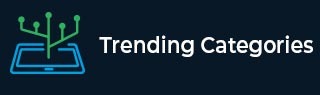
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Get the list of all the public methods in Java
A list of all the public methods of a class or interface that is represented by an object are provided using the method java.lang.Class.getMethods(). The public methods include that are declared by the class or interface and also those that are inherited by the class or interface.
Also, the getMethods() method returns a zero length array if the class or interface has no public methods or if a primitive type, array class or void is represented in the Class object.
A program that demonstrates this is given as follows −
Example
import java.lang.reflect.Method; public class Main { public static void main(String[] argv) throws Exception { Class c = java.lang.Thread.class; Method[] methods = c.getMethods(); System.out.println("The public methods of the java.lang.Thread class are:
"); for (int i = 0; i < methods.length; i++) { System.out.println(methods[i]); } } }
Output
The public methods of the java.lang.Thread class are: public void java.lang.Thread.run() public java.lang.String java.lang.Thread.toString() public boolean java.lang.Thread.isInterrupted() public static native java.lang.Thread java.lang.Thread.currentThread() public final java.lang.String java.lang.Thread.getName() public synchronized void java.lang.Thread.start() public final synchronized void java.lang.Thread.join(long,int) throws java.lang.InterruptedException public final synchronized void java.lang.Thread.join(long) throws java.lang.InterruptedException public final void java.lang.Thread.join() throws java.lang.InterruptedException public final java.lang.ThreadGroup java.lang.Thread.getThreadGroup() public java.lang.StackTraceElement[] java.lang.Thread.getStackTrace() public static native boolean java.lang.Thread.holdsLock(java.lang.Object) public final void java.lang.Thread.checkAccess() public static void java.lang.Thread.dumpStack() public static native void java.lang.Thread.yield() public final void java.lang.Thread.setPriority(int) public final void java.lang.Thread.setDaemon(boolean) public static native void java.lang.Thread.sleep(long) throws java.lang.InterruptedException public static void java.lang.Thread.sleep(long,int) throws java.lang.InterruptedException public final synchronized void java.lang.Thread.stop(java.lang.Throwable) public final void java.lang.Thread.stop() public void java.lang.Thread.interrupt() public static boolean java.lang.Thread.interrupted() public void java.lang.Thread.destroy() public final native boolean java.lang.Thread.isAlive() public final void java.lang.Thread.suspend() public final void java.lang.Thread.resume() public final int java.lang.Thread.getPriority() public final synchronized void java.lang.Thread.setName(java.lang.String) public static int java.lang.Thread.activeCount() public static int java.lang.Thread.enumerate(java.lang.Thread[]) public native int java.lang.Thread.countStackFrames() public final boolean java.lang.Thread.isDaemon() public java.lang.ClassLoader java.lang.Thread.getContextClassLoader() public void java.lang.Thread.setContextClassLoader(java.lang.ClassLoader) public static java.util.Map java.lang.Thread.getAllStackTraces() public long java.lang.Thread.getId() public java.lang.Thread$State java.lang.Thread.getState() public static void java.lang.Thread.setDefaultUncaughtExceptionHandler(java.lang.Thread$UncaughtExceptionHandler) public static java.lang.Thread$UncaughtExceptionHandler java.lang.Thread.getDefaultUncaughtExceptionHandler() public java.lang.Thread$UncaughtExceptionHandler java.lang.Thread.getUncaughtExceptionHandler() public void java.lang.Thread.setUncaughtExceptionHandler(java.lang.Thread$UncaughtExceptionHandler) public final void java.lang.Object.wait(long,int) throws java.lang.InterruptedException public final native void java.lang.Object.wait(long) throws java.lang.InterruptedException public final void java.lang.Object.wait() throws java.lang.InterruptedException public boolean java.lang.Object.equals(java.lang.Object) public native int java.lang.Object.hashCode() public final native java.lang.Class java.lang.Object.getClass() public final native void java.lang.Object.notify() public final native void java.lang.Object.notifyAll()
Advertisements