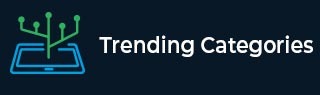
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Get the information about the memory layout of the masked array in Numpy
To get the information about the memory layout of the masked array, use the ma.MaskedArray.flags in Numpy. Masked arrays are arrays that may have missing or invalid entries. The numpy.ma module provides a nearly work-alike replacement for numpy that supports data arrays with masks.
A masked array is the combination of a standard numpy.ndarray and a mask. A mask is either nomask, indicating that no value of the associated array is invalid, or an array of booleans that determines for each element of the associated array whether the value is valid or not.
Steps
At first, import the required library −
import numpy as np import numpy.ma as ma
Create a numpy array using the numpy.array() method −
arr = np.array([[35, 85], [67, 33]]) print("Array...
", arr) print("
Array type...
", arr.dtype)
Get the dimensions of the Array −
print("Array Dimensions...",arr.ndim)
Get the information about the memory layout of the array −
print("
Array flags...
",arr.flags)
Create a masked array and mask some of them as invalid −
maskArr = ma.masked_array(arr, mask =[[0, 0], [ 0, 1]]) print("
Our Masked Array
", maskArr) print("
Our Masked Array type...
", maskArr.dtype)
Get the dimensions of the Masked Array −
print(" Our Masked Array Dimensions...",maskArr.ndim)
To get the information about the memory layout of the masked array, use the ma.MaskedArray.flags in Numpy −
print("
Our Masked Array flags...
",maskArr.flags)
Example
import numpy as np import numpy.ma as ma # Create a numpy array using the numpy.array() method arr = np.array([[35, 85], [67, 33]]) print("Array...
", arr) print("
Array type...
", arr.dtype) # Get the dimensions of the Array print("Array Dimensions...",arr.ndim) # Get the information about the memory layout of the array print("
Array flags...
",arr.flags) # Create a masked array and mask some of them as invalid maskArr = ma.masked_array(arr, mask =[[0, 0], [ 0, 1]]) print("
Our Masked Array
", maskArr) print("
Our Masked Array type...
", maskArr.dtype) # Get the dimensions of the Masked Array print(" Our Masked Array Dimensions...",maskArr.ndim) # To get the information about the memory layout of the masked array, use the ma.MaskedArray.flags in Numpy print("
Our Masked Array flags...
",maskArr.flags)
Output
Array... [[35 85] [67 33]] Array type... int64 Array Dimensions... 2 Array flags... C_CONTIGUOUS : True F_CONTIGUOUS : False OWNDATA : True WRITEABLE : True ALIGNED : True WRITEBACKIFCOPY : False UPDATEIFCOPY : False Our Masked Array [[35 85] [67 --]] Our Masked Array type... int64 Our Masked Array Dimensions... 2 Our Masked Array flags... C_CONTIGUOUS : True F_CONTIGUOUS : False OWNDATA : False WRITEABLE : True ALIGNED : True WRITEBACKIFCOPY : False UPDATEIFCOPY : False