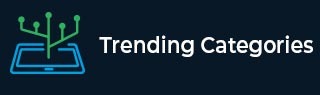
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Get key with maximum value in Dictionary in Python
A Python dictionary contains key value pairs. In this article we will see how to get the key of the element whose value is maximum in the given Python dictionary.
With max and get
We use the get function and the max function to get the key.
Example
dictA = {"Mon": 3, "Tue": 11, "Wed": 8} print("Given Dictionary:\n",dictA) # Using max and get MaxKey = max(dictA, key=dictA.get) print("The Key with max value:\n",MaxKey)
Output
Running the above code gives us the following result −
Given Dictionary: {'Mon': 3, 'Tue': 11, 'Wed': 8} The Key with max value: Tue
With itemgetter and max
With itemgetter function we get the items of the dictionary and by indexing it to position one we get the values. Next we apply why the max function and finally we get the required key.
Example
import operator dictA = {"Mon": 3, "Tue": 11, "Wed": 8} print("Given Dictionary:\n",dictA) # Using max and get MaxKey = max(dictA.items(), key = operator.itemgetter(1))[0] print("The Key with max value:\n",MaxKey)
Output
Running the above code gives us the following result −
Given Dictionary: {'Mon': 3, 'Tue': 11, 'Wed': 8} The Key with max value: Tue
Advertisements