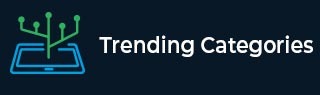
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Get key from value in JavaScript
In the given problem statement we have to write a function which will help to get the key of the given value with the usage of Javascript functionalities. So basically in Javascript if we want to access the keys or value from the given object we can access it by dot notation or bracket notation.
Understanding the problem statement
The problem statement says that we have to find a key from a value in a Javascript object. That means we are given a Javascript object and a value so we need to find the corresponding key which maps to that value in the object.
For example we have a Javascript object like
{ key1: 'value1', key2: 'value2', key3: value3, };
So we can see the value e.g value2 and we need to find its key so we can see here the key for value2 is key2.
Logic for the given problem
To solve the given problem we can iterate over the key value pairs in the object and then we will check if the current value matches the given value. If the value matches we can return the corresponding key of the given value. Suppose the value has not been matched in the given object then we have to return the output as null because there is no element like this in the data.
Algorithm
Step 1 − Define a function to get the key of the given value. So pass two parameters − appObj and value.
Step 2 − As we have to find out the key so to store the key we will declare a variable and initialize its value with the help of entries method and inside this function pass appObj and for this data we will find the match of the key and value.
Step 3 − Define a condition block to determine if the key object's value is equal to the actual value of the key. Then return the key if the condition is true.
Step 4 − If the given value is not retrieved then the value returned as null.
Code for the algorithm
//function for getting the key of the given value function keyByValue(appObj, value) { const [key] = Object.entries(appObj).find(([key, val]) => val === value) || []; return key || null; } const appObj = { Application1: 'Learning', Application2: 'Ecommerce', Application3: 'Software', Application4: 'Business', Application5: 'School', }; const value1 = 'Ecommerce'; const value2 = 'Business'; const key1 = keyByValue(appObj, value1); const key2 = keyByValue(appObj, value2); console.log(key1); console.log(key2);
Complexity
The time complexity of the approach is O(n) because we need to iterate over all the key value pairs in the worst case. And the space consumed by the algorithm is constant O(1). Because we are not using any additional data structures.
Conclusion
So for the above code we have used a for loop to search for a value in a Javascript object and get the corresponding key. This function has a time complexity of O(n) and space complexity of O(1).