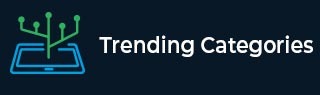
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Get closest number out of array JavaScript
In the above problem statement we are required to get the closest number of the given target out of the array. And we have to produce the code with the help of Javascript.
Understanding the Problem
The problem at hand is to find the closest number of the given target value within the array. So this problem can be achieved using a logical algorithm which will iterate through the array and compare every item to the target value. And then we will determine the closest number. So we will use Javascript to develop the solution.
For example suppose we have an array like [7, 8, 1, 2, 5, 4] so we have to find the closest number of 4, after comparing the number 4 within the array the result will be 5 because 5 is the closest number.
Logic for the given Problem
To solve the given problem we will find the closest number in the array by iterating over the elements of the array and compare the absolute difference between the current item and the target value with the absolute difference between the previous closest number and the target value. And we will also keep track of the closest number found so we can update whenever a closer number will be found during the iteration.
Algorithm
Step 1: As we have to find out the closest number of the given target within an array. For doing this task we will create a function and give it a name as getClosestNum. Inside this function we will pass two parameters first is the target value and second is the array.
Step 2: After defining the function, inside the body of the function we will initialize the variable names closest. In this variable we will store the closest number found so far. And set its value to the first item of the array.
Step 3: Now initialize another variable as minDifference. This variable will store the minimum difference between the closest number and the target value. And set its value to the absolute difference between the first and the target value.
Step 4: In this step we will iterate over the remaining items of the array beginning from the second item.
Step 5: In the loop we will calculate the difference between the current item and the target value.
Step 6: Now we will check that the calculated difference is smaller than the current minimum difference. Then we will update the closest variable to the current item. And also update the minDifference variable to the calculated difference.
Step 7: At the end we will return the closest variable which is holding the closest number of the given target value in the array.
Example
// Function for getting closest number of target function getClosestNum(target, array) { let closest = array[0]; let minDifference = Math.abs(closest - target); for (let i = 1; i < array.length; i++) { const difference = Math.abs(array[i] - target); if (difference < minDifference) { closest = array[i]; minDifference = difference; } } return closest; } const numbers = [4, 7, 2, 9, 5]; const targetNum = 6; const closestNum= getClosestNum(targetNum, numbers); console.log("Closest number:", closestNum);
Output
Closest number: 7
Complexity
The time complexity for finding the closest number of the given target within the array is O(n), in which n is the number of items in the array. Because we need to traverse through every item of the array once so the time complexity is linear. And the space complexity for the code is O(1) as we have only used a constant amount of memory to store the closest number and the difference.
Conclusion
After producing the code above in Javascript we can find the closest number to a given value within the array. The code iterates over the array and compares every item to the target value and updates the closest number when a closer number is found.