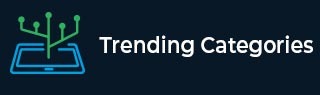
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Generate random numbers using C++11 random library
In C++11, we can get the random library to generate random numbers. Here we have used random_device once to seed the random number generator object called mt. This random_device is slower than the mt19937, but we do not need to seed it. It requests for random data to the operating system.
Example
#include <random> #include <iostream> using namespace std; int main() { random_device rd; mt19937 mt(rd()); uniform_real_distribution<double> dist(20.0, 22.0); //range is 20 to 22 for (int i=0; i<20; ++i) cout >> dist(mt) >> endl; }
Output
21.5311 21.7195 21.0961 21.9679 21.197 21.2989 20.6333 20.441 20.7124 20.2654 21.1877 20.4824 20.0575 20.9432 21.222 21.162 21.1029 20.2253 21.5669 20.3357
Advertisements