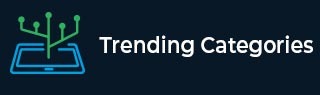
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Generate a Vandermonde matrix in Numpy
To generate a Vandermonde matrix, use the np.ma.vander() method in Python Numpy. A Vandermonde matrix, named after Alexandre-Théophile Vandermonde, is a matrix with the terms of a geometric progression in each row.
The columns of the output matrix are powers of the input vector. The order of the powers is determined by the increasing boolean argument. Specifically, when increasing is False, the i-th output column is the input vector raised element-wise to the power of N - i - 1. Such a matrix with a geometric progression in each row is named for Alexandre- Theophile Vandermonde.
Steps
At first, import the required library −
import numpy as np
Create an array with int elements using the numpy.array() method −
arr = np.array([93, 33, 76, 73, 88]) print("Array...
", arr)
Create a masked array and mask some of them as invalid −
maskArr = ma.masked_array(arr, mask =[0, 1, 0, 0, 1]) print("
Our Masked Array...
", maskArr)
Get the type of the masked array −
print("
Our Masked Array type...
", maskArr.dtype)
Get the dimensions of the Masked Array −
print("
Our Masked Array Dimensions...
",maskArr.ndim)
Get the shape of the Masked Array −
print("
Our Masked Array Shape...
",maskArr.shape)
Get the number of elements of the Masked Array −
print("
Number of elements in the Masked Array...
",maskArr.size)
To generate a Vandermonde matrix, use the np.ma.vander() method in Python Numpy −
print("
Result..
.", np.ma.vander(maskArr))
Example
import numpy as np import numpy.ma as ma # Create an array with int elements using the numpy.array() method arr = np.array([93, 33, 76, 73, 88]) print("Array...
", arr) # Create a masked array and mask some of them as invalid maskArr = ma.masked_array(arr, mask =[0, 1, 0, 0, 1]) print("
Our Masked Array...
", maskArr) # Get the type of the masked array print("
Our Masked Array type...
", maskArr.dtype) # Get the dimensions of the Masked Array print("
Our Masked Array Dimensions...
",maskArr.ndim) # Get the shape of the Masked Array print("
Our Masked Array Shape...
",maskArr.shape) # Get the number of elements of the Masked Array print("
Number of elements in the Masked Array...
",maskArr.size) # To generate a Vandermonde matrix, use the np.ma.vander() method in Python Numpy print("
Result..
.", np.ma.vander(maskArr))
Output
Array... [93 33 76 73 88] Our Masked Array... [93 -- 76 73 --] Our Masked Array type... int64 Our Masked Array Dimensions... 1 Our Masked Array Shape... (5,) Number of elements in the Masked Array... 5 Result.. . [[74805201 804357 8649 93 1] [ 0 0 0 0 0] [33362176 438976 5776 76 1] [28398241 389017 5329 73 1] [ 0 0 0 0 0]]