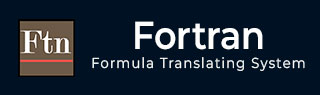
- Fortran Tutorial
- Fortran - Home
- Fortran - Overview
- Fortran - Environment Setup
- Fortran - Basic Syntax
- Fortran - Data Types
- Fortran - Variables
- Fortran - Constants
- Fortran - Operators
- Fortran - Decisions
- Fortran - Loops
- Fortran - Numbers
- Fortran - Characters
- Fortran - Strings
- Fortran - Arrays
- Fortran - Dynamic Arrays
- Fortran - Derived Data Types
- Fortran - Pointers
- Fortran - Basic Input Output
- Fortran - File Input Output
- Fortran - Procedures
- Fortran - Modules
- Fortran - Intrinsic Functions
- Fortran - Numeric Precision
- Fortran - Program Libraries
- Fortran - Programming Style
- Fortran - Debugging Program
- Fortran Resources
- Fortran - Quick Guide
- Fortran - Useful Resources
- Fortran - Discussion
Fortran - Reduction Functions
The following table describes the reduction functions:
Function | Description |
---|---|
all(mask, dim) | It returns a logical value that indicates whether all relations in mask are .true., along with only the desired dimension if the second argument is given. |
any(mask, dim) | It returns a logical value that indicates whether any relation in mask is .true., along with only the desired dimension if the second argument is given. |
count(mask, dim) | It returns a numerical value that is the number of relations in mask which are .true., along with only the desired dimension if the second argument is given. |
maxval(array, dim, mask) | It returns the largest value in the array array, of those that obey the relation in the third argument mask, if that one is given, along with only the desired dimension if the second argument dim is given. |
minval(array, dim, mask) | It returns the smallest value in the array array, of those that obey the relation in the third argument mask, if that one is given, along with only the desired dimension if the second argument DIM is given. |
product(array, dim, mask) | It returns the product of all the elements in the array array, of those that obey the relation in the third argument mask, if that one is given, along with only the desired dimension if the second argument dim is given. |
sum (array, dim, mask) | It returns the sum of all the elements in the array array, of those that obey the relation in the third argument mask, if that one is given, along with only the desired dimension if the second argument dim is given. |
Example
The following example demonstrates the concept:
program arrayReduction real, dimension(3,2) :: a a = reshape( (/5,9,6,10,8,12/), (/3,2/) ) Print *, all(a>5) Print *, any(a>5) Print *, count(a>5) Print *, all(a>=5 .and. a<10) end program arrayReduction
When the above code is compiled and executed, it produces the following result:
F T 5 F
Example
The following example demonstrates the concept:
program arrayReduction implicit none real, dimension(1:6) :: a = (/ 21.0, 12.0,33.0, 24.0, 15.0, 16.0 /) Print *, maxval(a) Print *, minval(a) Print *, sum(a) Print *, product(a) end program arrayReduction
When the above code is compiled and executed, it produces the following result:
33.0000000 12.0000000 121.000000 47900160.0
fortran_arrays.htm
Advertisements