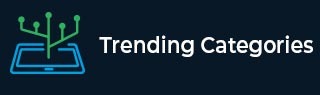
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Format floating point number in Java
Let’s say we have the following two values.
double val1 = 20.932; double val2 = 11.67;
Let us now format these floating-point numbers. Firstly, we are formatting euler’s number withMath.exp(). After that, we have also evaluated log. The %.3f you can see here is what we used for formatting the numbers.
System.out.printf("exp(%.3f) = %.3f%n", val1, Math.exp(val1)); System.out.printf("log = %.3f%n", val1, Math.log(val1));
The following is an example where we have also shown other ways to format float in Java.
Example
public class Demo { public static void main(String args[]) { double val1 = 20.932; double val2 = 11.67; System.out.format("%f%n", val1); System.out.printf("exp(%.3f) = %.3f%n", val1, Math.exp(val1)); System.out.printf("log = %.3f%n", val1, Math.log(val1)); System.out.format("%3f%n", val2); System.out.format("%+3f%n", val2); System.out.printf("pow(%.3f, %.3f) = %.3f%n", val1, val2, Math.pow(val1, val2)); } }
Output
20.932000 exp(20.932) = 1232117412.659 log = 20.932 11.670000 +11.670000 pow(20.932, 11.670) = 2593350237975675.500
Advertisements