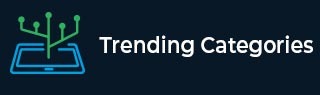
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
fma() function in C++
Given the task is to show the working of fma() function in C++. In this article we will look into what parameters this function need and what results it will be returning.
fma() is an inbuilt function of cmath header file, which accepts three parameters x, y and z and return the result x*y+z without losing the precision in any intermediate result.
Syntax
float fma(float x, float y, float z);
Or
double fma(double x, double y, double z);
Or
long double fma(long double x, long double y, long double z);
Parameters
x − The first element to be multiplied.
y − The second element with which x is to be multiplied.
z − Third element which will be added to the result of x and y.
Return value
The function returns the exact result of x*y+z.
Example
#include<iostream> #include<cmath> using namespace std; int main() { double x = 2.1, y = 4.2, z = 9.4, answer; answer = fma(x, y, z); cout << x << " * " << y << " + " << z << " = " << answer << endl; return 0; }
Output
If we run the above code it will generate the following output −
2.1 * 4.2 + 9.4 = 18.22
Example
#include<bits/stdc++.h> using namespace std; int main() { double a = 7.4, b = 9.3, c = 1.2; double ans = fma(a, b, c); cout << a << " * " << b << " + " << c << " = " << ans << endl; return 0; }
Output
If we run the above code it will generate the following output −
7.4 * 9.3 + 1.2 = 70.02
Advertisements