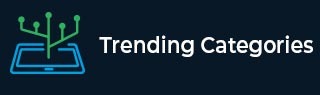
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Flip String to Monotone Increasing in C++
Suppose a string of '0's and '1's is given. That string will be monotonic increasing if it consists of some number of '0's (possibly 0), followed by some number of '1's (also possibly 0.). We have a string S of '0's and '1's, and we may flip any '0' to a '1' or a '1' to a '0'. Find the minimum number of flips to make S monotone increasing. So if the input is like “010110”, then the output will be 2. By flipping we can get “011111” or “000111”.
To solve this, we will follow these steps −
n := size of S, set flipCount := 0, oneCount := 0
for i in range 0 to n – 1
if S[i] is 0, then
if oneCount = 0, then skip to the next iteration
increase the flipCount by 1
otherwise increase oneCount by 1
if oneCount < flipCount, then set flipCount := oneCount
return flipCount
Let us see the following implementation to get better understanding −
Example
#include <bits/stdc++.h> using namespace std; class Solution { public: int minFlipsMonoIncr(string S) { int n = S.size(); int flipCount = 0; int oneCount = 0; for(int i = 0; i < n; i++){ if(S[i] == '0'){ if(oneCount == 0) continue; flipCount++; } else oneCount++; if(oneCount < flipCount) flipCount = oneCount; } return flipCount; } }; main(){ Solution ob; cout << (ob.minFlipsMonoIncr("010110")); }
Input
"010110"
Output
2