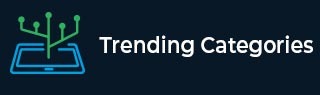
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Fixed Point in Python
Suppose we have an array A of unique integers sorted in ascending order, we have to return the smallest index i that satisfies A[i] == i. Return -1 if no such i exists. So if the array is like [-10,-5,0,3,7], then the output will be 3, as A[3] = 3 the output will be 3.
To solve this, we will follow these steps −
- For i in range 0 to length of A
- if i = A[i], then return i
- return -1
Example(Python)
Let us see the following implementation to get a better understanding −
class Solution(object): def fixedPoint(self, A): for i in range(len(A)): if i == A[i]: return i return -1 ob1 = Solution() print(ob1.fixedPoint([-10,-5,0,3,7]))
Input
[-10,-5,0,3,7]
Output
3
Advertisements