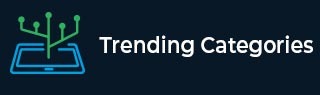
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
First Missing Positive in Python
Suppose we have one unsorted integer array; we have to find the smallest missing positive number. So if the array is like [4, -3, 1, -1], then the result will be 2.
To solve this, we will follow these steps −
set i := 0 and update array nums by adding one 0 before all numbers
for i in range 0 to length of nums
while nums[i] >= 0 and nums[i] < length of nums and nums[nums[i]] is not nums[i] −
nums[nums[i]] := nums[i]
nums[i] := nums[nums[i]]
num := 1
for i in range 1 to length of nums
if num = nums[i], then increase num by 1
return num
Example
Let us see the following implementation to get better understanding −
class Solution(object): def firstMissingPositive(self, nums): i = 0 nums = [0] + nums for i in range(len(nums)): while nums[i]>=0 and nums[i]<len(nums) and nums[nums[i]]!=nums[i]: nums[nums[i]],nums[i] = nums[i],nums[nums[i]] num = 1 for i in range(1,len(nums)): if num == nums[i]: num+=1 return num ob = Solution() print(ob.firstMissingPositive([4,-3,1,-1]))
Input
[4,-3,1,-1]
Output
2
Advertisements