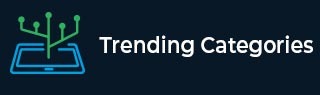
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Finding the missing number in an arithmetic progression sequence in JavaScript
Arithmetic Progression:
An arithmetic progression (AP) or arithmetic sequence is a sequence of numbers such that the difference between the consecutive terms is constant.
For instance, the sequence 5, 7, 9, 11, 13...
Suppose we have an array that represents elements of arithmetic progression in order. But somehow one of the numbers from the progression goes missing. We are required to write a JavaScript function that takes in one such array as the first and the only argument.
Then our function, in one iteration, should find and return the number which is missing from the sequence.
For example −
If the input array is −
const arr = [7, 13, 19, 31, 37, 43];
Then the output should be −
const output = 25;
because 25 is missing between 19 and 31
Example
The code for this will be −
const arr = [7, 13, 19, 31, 37, 43]; const findMissingNumber = (arr = []) => { let {length} = arr; let diff1 = arr[1] - arr[0]; let diff2 = arr[length - 1] - arr[length - 2]; if (diff1 !== diff2) { if (diff1 == 2 * diff2){ return arr[0] + diff2; }else{ return arr[length - 1] - diff1; }; }; for (let i = 1; i < length - 2; i++){ if (arr[i + 1] - arr[i] != diff1){ return arr[i] + diff1; }; }; return arr[0]; }; console.log(findMissingNumber(arr));
Output
And the output in the console will be −
25
Advertisements