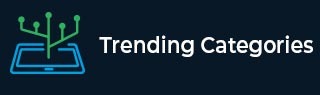
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Finding the count of total upside down numbers in a range using JavaScript
Upside Down Numbers
The numbers that remain the same when they’re rotated by 180 degrees are called upside down numbers.
For instance, 9116, 69.
Problem
We are required to write a JavaScript function that takes in a range array of two numbers. Our function should return the count of all the upside down numbers that fall in the specified range.
Example
Following is the code −
const range = [5, 125]; const flipNum = (number) => { const upsideDownDigits = [0, 1, -99, -99, -99, -99, 9, -99, 8, 6]; let reverseNumArr = String(number) .split('') .map(val => Number(val)) .reverse(); let flipDigitsNumArr = reverseNumArr.map(val => upsideDownDigits[val]); if (flipDigitsNumArr.includes(-99)) { return false; } let flipDigitsNum = Number( flipDigitsNumArr.reduce((accum, curr) => accum + String(curr)) ); return flipDigitsNum === number; }; const countUpsideDown = ([lowNum, highNum]) => { let uDNums = 0; for (let counter = lowNum; counter <= highNum; counter++) { uDNums += flipNum(counter) === true; } return uDNums; }; console.log(countUpsideDown(range));
Output
7
Advertisements