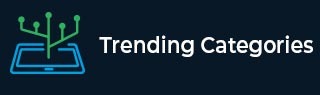
Data Structure
Networking
RDBMS
Operating System
Java
MS Excel
iOS
HTML
CSS
Android
Python
C Programming
C++
C#
MongoDB
MySQL
Javascript
PHP
Physics
Chemistry
Biology
Mathematics
English
Economics
Psychology
Social Studies
Fashion Studies
Legal Studies
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
- Who is Who
Finding product of Number digits in JavaScript
We are required to write a JavaScript program that takes in a number and finds the product of all of its digits.
Input output scenarios
Assume there is a number digit assigned to a variable and task is to find the product of the number.
Input = 12345; Output = 120
Let’s look into another scenario, where we convert the string number digit into int value and multiply them.
Input = "12345"; Output = 120
Math.floor()
Math.floor() function in JavaScript returns the largest integer which is less than equal to a given number. In simple words, this method rounds the number down to the nearest integer. Following is the syntax of the Math.floor() method −
Math.floor(x)
For a better understanding consider the following snippet, here we are providing various input values to this method.
Math.floor(10.95); // 10 Math.floor(10.05); // 10 Math.floor(10) // 10 Math.floor(-10.05); // -10 Math.floor(-10.95); // -1
Example 1
Product of Number digits
In this example below, we have a variable num holding 12345 we have created another variable product to store the product of the number (Initially set to 1).
When the num is not 0, the num will be divided by 10 and the result is stored product. Math.floor() is used here to eliminate the last digit.
<!DOCTYPE html> <html> <title>Product of number digits</title> <head> <p id = "para"></p> <script> var num = 12345; var product = 1; function func(){ while (num != 0) { product = product * (num % 10); num = Math.floor(num / 10); } document.getElementById("para").innerHTML = "The productduct of number digits is: " + product; }; func(); </script> </head> </html>
parseInt()
The parseInt() function will parse a string argument and return an integer value.
Syntax
Following is the syntax −
parseInt(string)
For a better understanding consider the following snippet, here we providing various input values to this method.
parseInt("20") // 20 parseInt("20.00") // 20 parseInt("20.23") // 20
Example
Converting string digit to integer
In the following example, we have defined a string holding an integer value 12345. Using for loops, we have iterated through each character of the string, converted them to integer values using the parseInt() method, and multiplied the resultant value with a variable (product: initialized with 1).
<!DOCTYPE html> <html> <head> <title>Product of Number digits</title> <button onClick = "func()">Click me! </button> <p id = "para"> </p> <script> function func(){ let string = "12345"; let product = 1; let n = string.length; for (let x = 0; x < n; x++){ product = product * (parseInt(string[x])); } document.getElementById("para").innerHTML = product; }; </script> </head> </html>
Example
By using a constructor
In this example, we have created a constructor of class and inside the constructor and we’ve used this keyword to call the immediate object later on we are taking input from the user by using the prompt() function. created another variable pro to store the product of the number. Initially set it to 1. When the value of the variable num is not 0, it will be divided by 10 and the result is stored in the variable pro. Here, we are using the Math.floor() method to eliminate the last digit.
<!DOCTYPE html> <html> <head> <title>Product of digits</title> </head> <body> <script> class Sum { constructor() { this.pro = 1; this.n = prompt("Enter a number:"); while (this.n != 0) { this.pro = this.pro * (this.n % 10); this.n = Math.floor(this.n / 10); } } } const res = new Sum(); document.write("Sum of Digit is = " + res.pro); </script> </body> </html>